用C语言写一个图书管理系统
时间: 2023-05-18 21:04:45 浏览: 74
好的,我可以为您提供一个简单的图书管理系统的代码示例,如下:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100
struct Book {
char title[50];
char author[50];
int id;
};
struct Library {
struct Book books[MAX_BOOKS];
int num_books;
};
void add_book(struct Library *lib, char *title, char *author, int id) {
if (lib->num_books >= MAX_BOOKS) {
printf("Error: library is full\n");
return;
}
struct Book book;
strcpy(book.title, title);
strcpy(book.author, author);
book.id = id;
lib->books[lib->num_books++] = book;
}
void print_books(struct Library *lib) {
printf("Library contents:\n");
for (int i = 0; i < lib->num_books; i++) {
printf("%d: %s by %s\n", lib->books[i].id, lib->books[i].title, lib->books[i].author);
}
}
int main() {
struct Library lib = {0};
add_book(&lib, "The Great Gatsby", "F. Scott Fitzgerald", 1);
add_book(&lib, "To Kill a Mockingbird", "Harper Lee", 2);
add_book(&lib, "1984", "George Orwell", 3);
print_books(&lib);
return 0;
}
这个程序使用了结构体来存储书籍的信息,并提供了添加书籍和打印图书馆内容的功能。您可以根据需要进行修改和扩展。
阅读全文
相关推荐




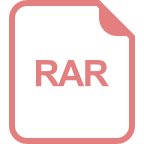





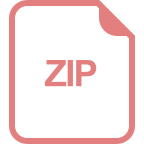
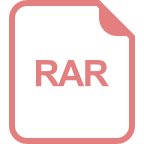
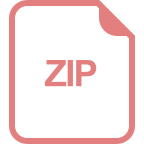
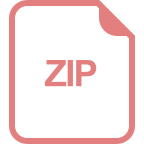