编写程序,输入一个字符串,统计其中大写字母、小写字母、数字及其他字符的个数,要求使用正则表达式。
时间: 2024-01-09 20:49:07 浏览: 122
以下是Python的代码示例:
```python
import re
string = input("请输入一个字符串:")
upper_count = len(re.findall(r'[A-Z]', string))
lower_count = len(re.findall(r'[a-z]', string))
digit_count = len(re.findall(r'\d', string))
other_count = len(re.findall(r'\W', string))
print("大写字母个数:", upper_count)
print("小写字母个数:", lower_count)
print("数字个数:", digit_count)
print("其他字符个数:", other_count)
```
代码中使用了re模块的findall()函数,以正则表达式匹配字符串中的大写字母、小写字母、数字和其他字符,并通过len()函数取得匹配结果的数量,最终输出各种字符的数量。
相关问题
编写程序,输入一个字符串,统计其中大写字母、小写字母、数字及其他字符的个数,要求使用正则表达式。第一行输入字符串,第二行输出统计结果(冒号为中交的冒号),每种类型之间用空格分隔。
对于这个问题,我可以回答。以下是代码示例:
import re
string = input()
uppercase_count = len(re.findall(r'[A-Z]', string))
lowercase_count = len(re.findall(r'[a-z]', string))
digit_count = len(re.findall(r'\d', string))
other_count = len(re.findall(r'[^A-Za-z\d\s]', string))
print("大写字母数量:", uppercase_count, ";小写字母数量:", lowercase_count, ";数字数量:", digit_count, ";其他字符数量:", other_count)
在JS中编写输入一个字符串,分别计算其中小写字母、大写字母、数字、其它字符的个数。
在JavaScript中,你可以使用正则表达式结合`String.prototype.match()`方法来统计字符串中的各种字符类型。下面是一个简单的函数示例:
```javascript
function countCharacters(str) {
var lowerCase = str.match(/[a-z]/g) ? str.match(/[a-z]/g).length : 0;
var upperCase = str.match(/[A-Z]/g) ? str.match(/[A-Z]/g).length : 0;
var numbers = str.match(/\d/g) ? str.match(/\d/g).length : 0;
var others = str.length - (lowerCase + upperCase + numbers);
return {
lowercase: lowerCase,
uppercase: upperCase,
digits: numbers,
other: others
};
}
// 使用示例
let inputStr = "HelloWorld123!@#$";
let result = countCharacters(inputStr);
console.log(result);
// 输出:{ lowercase: 9, uppercase: 2, digits: 3, other: 2 }
阅读全文
相关推荐
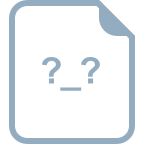




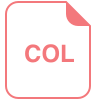






