java项目启动,需要将msg_info这个表的 词条加载到redis,key+object的形式
时间: 2023-11-27 11:55:14 浏览: 78
可以按照以下步骤实现:
1. 连接redis服务端,可以使用Jedis等redis客户端库。
2. 从数据库中查询msg_info表中的所有词条。
3. 遍历所有词条,将每个词条作为value,将key和value一起存入redis中,可以使用jedis的hset或hmset方法。
代码示例:
```java
Jedis jedis = new Jedis("localhost", 6379);
String sql = "select * from msg_info";
ResultSet rs = statement.executeQuery(sql);
while (rs.next()) {
String key = rs.getString("key");
String value = rs.getString("value");
jedis.hset("msg_info", key, value);
}
```
这段代码会将msg_info表中的所有词条存入redis中,以msg_info为key,以每个词条的key和value作为hash map中的key和value存储。
相关问题
springboot项目启动,需要将msg_info这个表的 词条加载到redis,key+object的形式
可以使用Spring Boot提供的注解来实现将msg_info表的词条加载到Redis中。具体步骤如下:
1. 在pom.xml文件中引入Redis依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
2. 在Spring Boot配置文件中配置Redis连接信息:
```
spring.redis.host=localhost
spring.redis.port=6379
spring.redis.password=
```
3. 定义RedisTemplate,用于操作Redis:
```java
@Configuration
public class RedisConfig {
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<String, Object> redisTemplate = new RedisTemplate<>();
redisTemplate.setConnectionFactory(redisConnectionFactory);
redisTemplate.setKeySerializer(new StringRedisSerializer());
redisTemplate.setValueSerializer(new GenericJackson2JsonRedisSerializer());
return redisTemplate;
}
}
```
4. 定义一个Service,用于从数据库中查询msg_info表的词条,并将其缓存到Redis中:
```java
@Service
public class MsgInfoService {
@Autowired
private RedisTemplate<String, Object> redisTemplate;
@Autowired
private MsgInfoMapper msgInfoMapper;
public void loadMsgInfoToRedis() {
List<MsgInfo> msgInfoList = msgInfoMapper.selectAll();
for (MsgInfo msgInfo : msgInfoList) {
String key = "msg_info:" + msgInfo.getId();
redisTemplate.opsForValue().set(key, msgInfo);
}
}
}
```
5. 在Spring Boot启动类中调用loadMsgInfoToRedis方法:
```java
@SpringBootApplication
public class Application implements CommandLineRunner {
@Autowired
private MsgInfoService msgInfoService;
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Override
public void run(String... args) throws Exception {
msgInfoService.loadMsgInfoToRedis();
}
}
```
这样,在Spring Boot启动时会自动将msg_info表的词条加载到Redis中,并以key+object的形式存储。可以使用redisTemplate操作Redis获取缓存的数据。
阅读全文
相关推荐
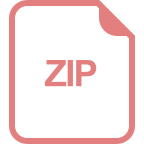
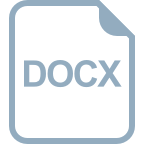
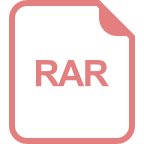
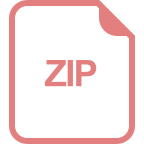
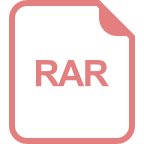
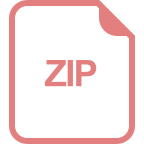
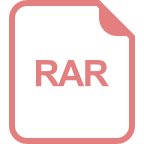
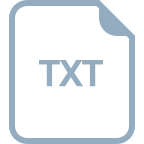
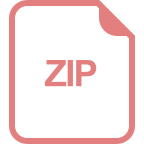
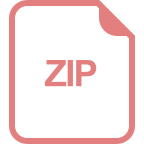
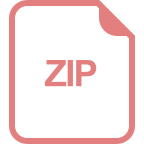
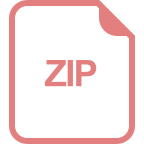
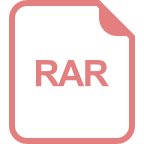
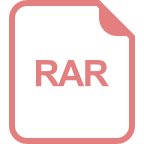