在文本文件中读取所有学生信息,每行为一个学生信息,包括学号、姓名
时间: 2024-05-14 21:16:14 浏览: 88
和成绩,以逗号分隔,如下所示:
```
001,张三,90
002,李四,80
003,王五,85
```
可以使用Python中的文件操作函数来读取文本文件中的数据。具体操作如下:
```python
with open('students.txt', 'r') as f:
for line in f:
# 去除行末的换行符
line = line.strip()
# 以逗号分隔学生信息
student = line.split(',')
# 输出学生信息
print('学号:{0},姓名:{1},成绩:{2}'.format(student[0], student[1], student[2]))
```
其中,`with open('students.txt', 'r') as f:` 表示打开名为 `students.txt` 的文件,并且以只读模式打开,`for line in f:` 表示遍历文件的每一行,`line.strip()` 表示去除每行末尾的换行符,`line.split(',')` 表示以逗号为分隔符,将每行的学生信息分割成一个列表并赋值给 `student` 变量,`print(...)` 表示输出学生信息。
相关问题
JAVA在文本文件中读取所有学生信息,每行为一个学生信息,包括学号、姓名
可以使用Java中的File类和Scanner类来读取文本文件中的数据。
假设文本文件中每行的格式为"学号 姓名",可以按照以下步骤读取所有学生信息:
1. 创建一个File对象,指定要读取的文本文件的路径。
```java
File file = new File("student.txt");
```
2. 创建一个Scanner对象,读取文本文件中的数据。
```java
Scanner scanner = new Scanner(file);
```
3. 使用while循环读取每行数据,直到文件结束。
```java
while (scanner.hasNextLine()) {
// 读取一行数据
String line = scanner.nextLine();
// 解析学号和姓名
String[] parts = line.split(" ");
String id = parts[0];
String name = parts[1];
// TODO: 处理学生信息
}
```
在每次循环中,可以使用String的split()方法将一行数据按照空格分割成学号和姓名两部分。
然后,可以根据需要对学生信息进行处理,例如将它们存储到一个List或Map中。
完整代码示例:
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class ReadStudentInfo {
public static void main(String[] args) throws FileNotFoundException {
File file = new File("student.txt");
Scanner scanner = new Scanner(file);
List<Student> students = new ArrayList<>();
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] parts = line.split(" ");
String id = parts[0];
String name = parts[1];
Student student = new Student(id, name);
students.add(student);
}
System.out.println("读取到的学生信息:");
for (Student student : students) {
System.out.println(student);
}
}
static class Student {
String id;
String name;
public Student(String id, String name) {
this.id = id;
this.name = name;
}
@Override
public String toString() {
return "Student{" +
"id='" + id + '\'' +
", name='" + name + '\'' +
'}';
}
}
}
```
2.一个文本文件data1.txt,其内容为学生信息,每一行为一个信息,包含学号、姓名、数学成绩、英语成绩、物理成绩,数据之间以空白字符分隔。编程从该文件中读入这些信息,统计学生人数并求出每门成绩的平均分,最高分和最低分,在result.txt 中写入学生人数并求出每门成绩的平均分,最高分和最低分。C++C++
下面是一个简单的 C++ 实现:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
struct student {
string id;
string name;
int math;
int english;
int physics;
};
int main() {
ifstream fin("data1.txt");
if (!fin) {
cerr << "Cannot open file!" << endl;
return -1;
}
vector<student> students;
string line;
while (getline(fin, line)) {
student s;
stringstream ss(line);
ss >> s.id >> s.name >> s.math >> s.english >> s.physics;
students.push_back(s);
}
int count = students.size();
double math_sum = 0.0;
double english_sum = 0.0;
double physics_sum = 0.0;
int math_max = -1;
int english_max = -1;
int physics_max = -1;
int math_min = 101;
int english_min = 101;
int physics_min = 101;
for (auto s : students) {
math_sum += s.math;
english_sum += s.english;
physics_sum += s.physics;
math_max = max(math_max, s.math);
english_max = max(english_max, s.english);
physics_max = max(physics_max, s.physics);
math_min = min(math_min, s.math);
english_min = min(english_min, s.english);
physics_min = min(physics_min, s.physics);
}
double math_avg = math_sum / count;
double english_avg = english_sum / count;
double physics_avg = physics_sum / count;
ofstream fout("result.txt");
fout << "Number of students: " << count << endl;
fout << "Math average: " << math_avg << " Max: " << math_max << " Min: " << math_min << endl;
fout << "English average: " << english_avg << " Max: " << english_max << " Min: " << english_min << endl;
fout << "Physics average: " << physics_avg << " Max: " << physics_max << " Min: " << physics_min << endl;
fin.close();
fout.close();
return 0;
}
```
首先,我们打开文件并读取每一行,将每个学生的信息存储在一个结构体中,然后将所有学生存储在一个 vector 中。接下来,我们可以使用 for 循环来计算每个学生的成绩总分以及每个科目的最高分和最低分。最后,我们计算每个科目的平均分,并将结果写入另一个文件中。
阅读全文
相关推荐
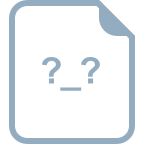
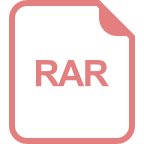




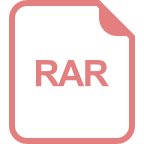
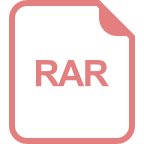
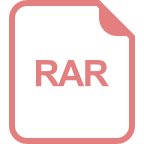
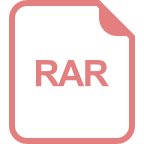
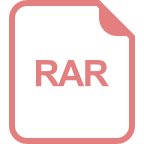
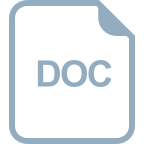
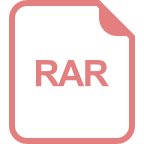
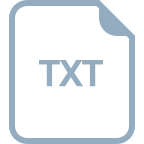
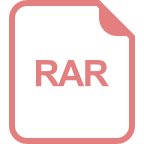
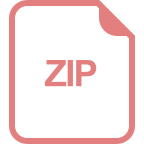