Explain the difference between routing, forwarding, and switching.用中文解释
时间: 2024-05-21 15:11:42 浏览: 25
路由、转发和交换的区别在于它们所处理的网络层级不同。路由是对大规模网络不同部分之间的数据包进行转移的过程,是在网络层中实现的;转发是将从源端传来的数据包当场向目的网络接口进行发送的过程,是在数据链路层中实现的;交换则是在物理层和数据链路层中实现的,它是将数据包从一个接口直接发送到另一个接口,无需传递到网络层。简单来说,路由用于连接多个网络;转发用于在同一个网络中传递数据;交换用于在同一个局域网中传递数据。
相关问题
Explain the difference between routing, forwarding, and switching.
Sure! Routing is the process of determining the best path for network traffic to reach its destination. Forwarding is the act of sending the data packets along that determined path. Switching, on the other hand, happens at a smaller scale, within local networks. In switching, data packets are received and then forwarded to the appropriate port that connects to the network where the destination device is located.
explain the difference between "for" and "generate for" in Verilog
### 回答1:
"for" 和 "generate for" 在 Verilog 中有着明显的区别。
"for" 是一个循环控制语句,用于在给定范围内重复执行语句。它通常在程序流程控制中使用,例如,在实现循环结构时。
"generate for" 是 Verilog 中的一个结构体化生成语句,用于生成一组重复的模块或电路元素。它可以通过设置生成的数量来动态生成模块,并且可以在生成过程中改变模块的参数,以实现更高级的功能。
简而言之,"for" 是程序控制语句,而 "generate for" 是模块生成语句,在生成多个相似的模块或电路元素时特别有用。
### 回答2:
在Verilog语言中,"for"和"generate for"是两种不同的循环结构。
首先,"for"(一般循环)是一种用于在模块或过程中重复执行代码块的结构。它类似于其他编程语言中的传统循环,通过指定一个循环变量、起始值、结束条件和步长来控制循环。例如,下面的代码展示了一个使用"for"循环输出数字0到9的例子:
```
integer i;
for (i = 0; i < 10; i = i + 1) begin
$display("i is %d", i);
end
```
在这个例子中,循环变量i从0开始,每次循环增加1,直到i小于10停止。每次循环,都会执行被包含在循环体内的代码块。因此,程序将输出数字0到9。
另一方面,"generate for"是一种用于在编译时生成硬件结构的循环结构。它主要用于生成重复的硬件实例或生成其他结构性的代码。生成结构通常用于设计中重复的功能单元,例如多个寄存器或多路选择器。
在生成结构中,循环变量的值不是在运行时确定的,而是在编译时根据生成循环的范围计算得出的。生成循环类似于for循环,但它不会生成可执行的运行时代码。下面是一个使用"generate for"循环生成8个寄存器的例子:
```
genvar i;
generate
for (i = 0; i < 8; i = i + 1) begin: REG_GEN
reg [7:0] reg_inst;
end
endgenerate
```
在这个例子中,生成循环会根据循环变量i的范围,生成8个名称为REG_GEN的寄存器。这些寄存器将在实现时被实例化,但实例化的具体数量将在编译时确定。
总结而言,"for"循环用于在运行时重复执行代码块,而"generate for"循环用于在编译时重复生成硬件结构。这两种循环结构在Verilog语言中有不同的应用场景和语义。
### 回答3:
"for" 和 "generate for" 在 Verilog 中的区别可以从以下几个方面来解释。
1. 使用范围不同:在 Verilog 中,"for" 是用于在 always 块或 initial 块内部生成循环语句,用于在运行时多次执行相同的操作。而 "generate for" 是用于在模块中生成多个实例或定义多个变量等,用于在编译时生成硬件结构。
2. 作用域不同: "for" 的作用域是在运行时,只在 always 块或 initial 块内部有效。而 "generate for" 的作用域是在编译时,可以在整个模块中有效。
3. 控制方式不同: "for" 循环是通过条件或计数器来控制循环次数或循环终止条件。而 "generate for" 是通过参数或常量来控制生成的实例或变量的数量。
4. 主要用途不同: "for" 主要用于在模拟仿真环境下执行循环操作,如数据处理、状态更新等。而 "generate for" 主要用于在编译时生成多个实例或变量,如生成多个 FIFO 实例、多个状态机等。
总的来说,"for" 主要是在运行时控制具体的操作逻辑,而 "generate for" 主要是在编译时生成硬件结构。它们的使用方式和作用域不同,适用于不同的应用场景。
相关推荐
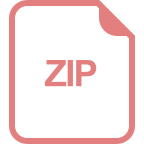
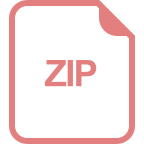
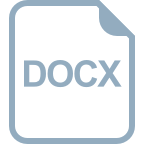












