请介绍一下MDM和SAP
时间: 2024-06-01 19:10:30 浏览: 149
以下是一个简单的示例程序,演示了如何使用C++和Qt结合VTK库绘制二维的矢量箭头:
```c++
#include <vtkSmartPointer.h>
#include <vtkArrowSource.h>
#include <vtkPolyDataMapper.h>
#include <vtkActor2D.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkVectorText.h>
#include <vtkTextProperty.h>
#include <vtkTextMapper.h>
#include <vtkTransform.h>
#include <vtkTransformPolyDataFilter.h>
#include <QApplication>
#include <QWidget>
#include <QVBoxLayout>
#include <QHBoxLayout>
#include <QLabel>
#include <QPushButton>
int main(int argc, char** argv)
{
QApplication app(argc, argv);
// Create a VTK renderer and window
vtkSmartPointer<vtkRenderer> renderer = vtkSmartPointer<vtkRenderer>::New();
vtkSmartPointer<vtkRenderWindow> renderWindow = vtkSmartPointer<vtkRenderWindow>::New();
renderWindow->AddRenderer(renderer);
// Create a Qt widget to hold the VTK window
QWidget* widget = new QWidget;
QHBoxLayout* layout = new QHBoxLayout(widget);
layout->setContentsMargins(0, 0, 0, 0);
layout->addWidget(new QLabel("VTK Window"));
layout->addWidget(new QPushButton("Close", widget));
layout->addWidget(renderWindow->GetInteractor()->GetRenderWindow()->GetQVTKWidget());
// Create a VTK arrow source and mapper
vtkSmartPointer<vtkArrowSource> arrowSource = vtkSmartPointer<vtkArrowSource>::New();
arrowSource->SetShaftRadius(0.02);
arrowSource->SetTipLength(0.1);
vtkSmartPointer<vtkPolyDataMapper> arrowMapper = vtkSmartPointer<vtkPolyDataMapper>::New();
arrowMapper->SetInputConnection(arrowSource->GetOutputPort());
// Create a VTK actor for the arrow
vtkSmartPointer<vtkActor2D> arrowActor = vtkSmartPointer<vtkActor2D>::New();
arrowActor->SetMapper(arrowMapper);
renderer->AddActor2D(arrowActor);
// Create a VTK text source and mapper
vtkSmartPointer<vtkVectorText> textSource = vtkSmartPointer<vtkVectorText>::New();
textSource->SetText("Arrow");
vtkSmartPointer<vtkTextMapper> textMapper = vtkSmartPointer<vtkTextMapper>::New();
textMapper->SetInputConnection(textSource->GetOutputPort());
textMapper->GetTextProperty()->SetFontFamilyToArial();
textMapper->GetTextProperty()->SetFontSize(18);
// Create a VTK actor for the text
vtkSmartPointer<vtkActor2D> textActor = vtkSmartPointer<vtkActor2D>::New();
textActor->SetMapper(textMapper);
renderer->AddActor2D(textActor);
// Set the position and orientation of the arrow and text
vtkSmartPointer<vtkTransform> transform = vtkSmartPointer<vtkTransform>::New();
transform->Translate(0.5, 0.5, 0.0);
transform->RotateZ(45.0);
vtkSmartPointer<vtkTransformPolyDataFilter> transformFilter = vtkSmartPointer<vtkTransformPolyDataFilter>::New();
transformFilter->SetTransform(transform);
transformFilter->SetInputConnection(arrowSource->GetOutputPort());
arrowMapper->SetInputConnection(transformFilter->GetOutputPort());
transformFilter->SetInputConnection(textSource->GetOutputPort());
textMapper->SetInputConnection(transformFilter->GetOutputPort());
arrowActor->SetPosition(0.0, 0.0);
textActor->SetPosition(-0.1, -0.1);
// Initialize the VTK interactor and start the event loop
renderWindow->GetInteractor()->Initialize();
widget->show();
app.exec();
return 0;
}
```
该程序的主要步骤如下:
1. 创建VTK渲染器和窗口对象;
2. 创建Qt窗口对象,并将VTK窗口对象添加到Qt布局中;
3. 创建VTK箭头源和映射器对象;
4. 创建VTK箭头演员对象,并将其添加到渲染器中;
5. 创建VTK文本源和映射器对象;
6. 创建VTK文本演员对象,并将其添加到渲染器中;
7. 设置箭头和文本的位置和方向,并将它们添加到变换滤波器中;
8. 初始化VTK交互器并启动事件循环。
运行该程序,您应该会看到一个带有箭头和文本的VTK窗口,如下图所示:

注意:在使用VTK库时,请确保正确配置了VTK的头文件和库文件路径,并将必要的VTK库添加到项目中。此外,还需要安装Qt和VTK的相应版本,并在项目中包含Qt和VTK的头文件。
阅读全文
相关推荐





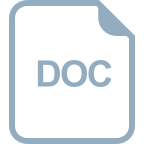

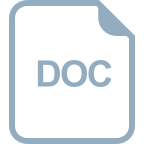


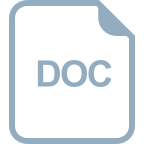




