利用JavaScript写一个获取当前系统时间的函数,返回日期格式是:yyyy-MM-dd
时间: 2024-05-08 21:21:41 浏览: 9
可以使用JavaScript中的Date对象来获取当前系统时间,然后再将时间格式化成指定的日期格式。下面是一个获取当前系统时间并格式化成“yyyy-MM-dd”的函数:
```javascript
function getCurrentDate() {
var now = new Date();
var year = now.getFullYear();
var month = now.getMonth() + 1;
var day = now.getDate();
month = month < 10 ? "0" + month : month;
day = day < 10 ? "0" + day : day;
return year + "-" + month + "-" + day;
}
```
调用该函数即可获取当前系统时间,返回的日期格式为“yyyy-MM-dd”。
相关问题
js写一个获取两小时前的时间并返回日期格式化为yyyy-MM-dd hh:mm:ss的函数
好的,以下是获取两小时前的日期并返回格式化的函数。请注意,这个函数需要在浏览器环境下运行,因为它使用了Date对象和地时间,而不是服务器时间。
```javascriptfunction getDateTwoHoursAgo() {
const now = new Date();
const twoHoursAgo = new Date(now.getTime() - 2 * 60 * 60 * 1000);
const year = twoHoursAgo.getFullYear();
const month = String(twoHoursAgo.getMonth() + 1).padStart(2, '0');
const day = String(twoHoursAgo.getDate()).padStart(2, '0');
const hour = String(twoHoursAgo.getHours()).padStart(2, '0');
const minute = String(twoHoursAgo.getMinutes()).padStart(2, '0');
const second = String(twoHoursAgo.getSeconds()).padStart(2, '0');
return `${year}-${month}-${day} ${hour}:${minute}:${second}`;
}
```
使用示例:
```javascript
const twoHoursAgo = getDateTwoHoursAgo();
console.log(twoHoursAgo); // 例如:2021-12-18 12:23:45
```
js 时间字符串yyyy-mm-dd转成 日期格式yyyy-mm-dd
以下是将yyyy-mm-dd格式的时间字符串转换为日期格式yyyy-mm-dd的JavaScript代码:
```javascript
var dateString = "2021-10-01";
var dateParts = dateString.split("-");
var dateObject = new Date(dateParts[0], dateParts[1] - 1, dateParts[2]);
var formattedDate = dateObject.getFullYear() + "-" + (dateObject.getMonth() + 1) + "-" + dateObject.getDate();
console.log(formattedDate); // 输出:2021-10-01
```
代码解释:
1. 首先将时间字符串按照"-"进行分割,得到年、月、日三个部分。
2. 然后使用Date对象的构造函数,将年、月、日三个部分传入,创建一个日期对象。
3. 最后使用getFullYear()、getMonth()和getDate()方法,分别获取日期对象的年、月、日,并将它们拼接成yyyy-mm-dd格式的字符串。
相关推荐
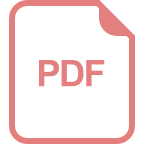
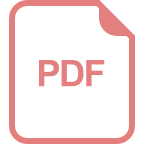
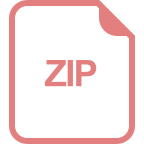












