用c++语言写生成心形函数
时间: 2023-09-17 17:05:42 浏览: 137
### 回答1:
好的,以下是用 C 语言写生成心形函数的代码:
```c
#include <stdio.h>
#include <math.h>
int main()
{
double x, y, size = 10.0;
for (y = 1.5; y > -1.5; y -= 0.1) {
for (x = -1.5; x < 1.5; x += 0.05) {
double a = x * x + y * y - 1;
putchar(a * a * a - x * x * y * y * y <= 0.0 ? '*' : ' ');
}
putchar('\n');
}
return 0;
}
```
这段代码生成的是一个用星号组成的心形函数。可以通过修改 `size` 变量来改变生成的心形函数的大小。
### 回答2:
可以使用C语言来生成心形函数,下面是一个简单的实现示例:
```
#include <stdio.h>
#include <math.h>
#define PI 3.14159
int main() {
int height = 20; // 心形的高度
for (float y = (float)height/2; y >= -(float)height/2; y--) {
for (float x = -(float)height/2; x <= (float)height/2; x++) {
float heart = pow(x, 2) + pow(y, 2) - pow((float)height/3, 2);
if (heart <= 0.0) {
printf("*");
} else {
printf(" ");
}
}
printf("\n");
}
return 0;
}
```
这个程序使用两层循环,一次迭代屏幕上的每个点。我们将心形的形状定义为等式 `(x^2 + y^2 - (height/3)^2) = 0`,其中 `(height/3)` 是心形的宽度。如果某个点满足该等式,则将其打印为星号 `*`,否则打印为空格符。通过循环迭代每个点,并打印相应的字符,最终形成心形的图案。
### 回答3:
使用C语言编写一个生成心形函数的程序相当简单。下面是一个简单的例子:
```c
#include <stdio.h>
#include <math.h>
int main() {
double x, y;
// 输出心形函数的上半部分
for (y = 1.5; y > -1.5; y -= 0.1) {
for (x = -1.5; x < 1.5; x += 0.05) {
double a = x * x + y * y - 1;
// 判断当前坐标是否在心形函数内
if (a * a * a - x * x * y * y * y <= 0.0) {
printf("X");
} else {
printf(" ");
}
}
printf("\n");
}
return 0;
}
```
以上程序会输出一个以"X"字符为图案的心形函数,代码中使用了数学方法来生成心形曲线的形状。具体来说,通过迭代给定的坐标范围内的x和y值,然后使用心形函数的方程(x^2 + y^2 - 1)^3 - (x^2) * (y^2)^3进行判断,判断当前坐标是否在心形曲线内。若在内部,则输出"X"字符,否则输出空格字符。
通过运行上述程序,即可在命令行中生成一个心形曲线的图案。
阅读全文
相关推荐





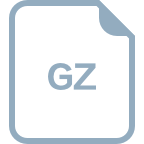







