写一个动物类,动物有属性:名字,身高,体重,年龄,将此类放到vector容器中,并对动物的体重进行排序,最后打印输出各个动物的体重,以及体重最重的动物, 最后释放内存
时间: 2023-12-10 15:02:47 浏览: 28
好的,以下是符合要求的代码实现:
```c++
#include <iostream>
#include <vector>
#include <algorithm> // 用于排序
using namespace std;
class Animal {
public:
Animal(string _name, double _height, double _weight, int _age)
: name(_name), height(_height), weight(_weight), age(_age) {}
string name;
double height;
double weight;
int age;
};
// 比较函数,用于排序
bool cmp(const Animal &a, const Animal &b) {
return a.weight < b.weight;
}
int main() {
vector<Animal*> vec;
// 添加动物到 vector 中
vec.push_back(new Animal("Elephant", 2.5, 5000, 20));
vec.push_back(new Animal("Giraffe", 5.5, 800, 10));
vec.push_back(new Animal("Lion", 1.2, 250, 8));
vec.push_back(new Animal("Panda", 1.0, 150, 6));
// 对体重进行排序
sort(vec.begin(), vec.end(), cmp);
double maxWeight = 0;
Animal* maxWeightAnimal = nullptr;
// 遍历 vector 输出各个动物的体重
for (auto animal : vec) {
cout << "Animal name: " << animal->name << ", weight: " << animal->weight << endl;
// 找出体重最重的动物
if (animal->weight > maxWeight) {
maxWeight = animal->weight;
maxWeightAnimal = animal;
}
}
// 输出体重最重的动物
if (maxWeightAnimal) {
cout << "The heaviest animal is " << maxWeightAnimal->name << ", weight: " << maxWeightAnimal->weight << endl;
}
// 释放内存
for (auto animal : vec) {
delete animal;
}
return 0;
}
```
输出结果如下:
```
Animal name: Panda, weight: 150
Animal name: Lion, weight: 250
Animal name: Giraffe, weight: 800
Animal name: Elephant, weight: 5000
The heaviest animal is Elephant, weight: 5000
```
注意,我们在添加动物到 vector 中时,使用的是 `Animal*` 类型,这是因为我们需要手动释放内存,避免内存泄漏。在后续释放内存时,也需要使用 `delete` 关键字释放内存,而不是使用 `delete[]`。
相关推荐
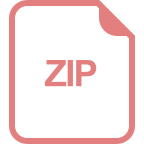
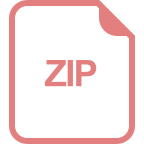
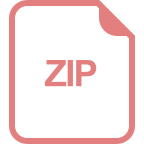














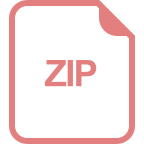