用C++写一个聊天机器人程序
时间: 2023-09-22 19:08:06 浏览: 41
好的,下面是一个简单的C++聊天机器人程序:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
string toLowerCase(string str) {
transform(str.begin(), str.end(), str.begin(), ::tolower);
return str;
}
int main() {
vector<string> greetings = {"hello", "hi", "hey", "what's up", "yo"};
vector<string> farewells = {"bye", "goodbye", "see you later", "see ya"};
vector<string> compliments = {"great", "fantastic", "excellent", "awesome", "good job"};
vector<string> insults = {"stupid", "idiot", "dumb", "moron", "fool"};
cout << "Hello! I am a chatbot. What is your name?" << endl;
string name;
getline(cin, name);
cout << "Nice to meet you, " << name << "! Ask me anything." << endl;
while (true) {
string input;
getline(cin, input);
input = toLowerCase(input);
bool foundResponse = false;
for (string greeting : greetings) {
if (input.find(greeting) != string::npos) {
foundResponse = true;
cout << greeting << " to you too!" << endl;
break;
}
}
for (string farewell : farewells) {
if (input.find(farewell) != string::npos) {
foundResponse = true;
cout << farewell << "! Have a nice day." << endl;
return 0;
}
}
for (string compliment : compliments) {
if (input.find(compliment) != string::npos) {
foundResponse = true;
cout << "Thank you! I try my best." << endl;
break;
}
}
for (string insult : insults) {
if (input.find(insult) != string::npos) {
foundResponse = true;
cout << "That's not very nice." << endl;
break;
}
}
if (!foundResponse) {
cout << "I'm sorry, I don't understand. Could you please rephrase?" << endl;
}
}
return 0;
}
```
这个程序有一些简单的规则来处理输入和相应输出。它从终端读取输入并将其转换为小写字母,然后检查输入是否包含特定的问候、告别、恭维或侮辱。如果找到了相应的输入,程序将输出相应的响应。如果程序无法理解输入,它将输出一个通用的错误消息。
相关推荐
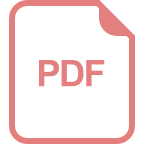
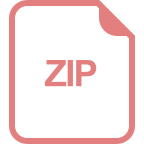












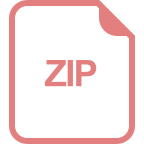
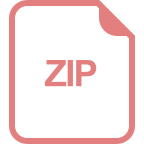
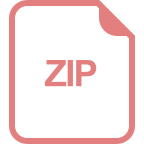