for循环怎么同时遍历x,y要两个一起变
时间: 2024-03-24 11:41:33 浏览: 7
可以使用C++11引入的范围for循环(range-based for loop)来同时遍历两个容器或数组,例如:
```c++
#include <iostream>
#include <vector>
int main() {
std::vector<int> x = {1, 2, 3};
std::vector<int> y = {4, 5, 6};
for (auto &a : x) {
for (auto &b : y) {
std::cout << "(" << a << ", " << b << ")" << std::endl;
}
}
return 0;
}
```
输出结果为:
```
(1, 4)
(1, 5)
(1, 6)
(2, 4)
(2, 5)
(2, 6)
(3, 4)
(3, 5)
(3, 6)
```
其中,`auto`关键字可以自动推导变量的类型,`&`表示使用引用方式遍历容器,可以避免在循环体内对容器元素进行拷贝。这里的两个for循环可以实现同时遍历两个容器x和y的功能。
相关问题
for循环怎么同时遍历x,y要两个一起变,x和y要一起加不同的数
可以使用C++11引入的范围for循环(range-based for loop)来同时遍历两个容器或数组,并且结合`std::transform()`算法来实现对两个容器的同时操作,例如:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> x = {1, 2, 3};
std::vector<int> y = {4, 5, 6};
std::vector<int> z(x.size());
std::transform(x.begin(), x.end(), y.begin(), z.begin(),
[](int a, int b) { return a + b + 1; });
for (auto &a : z) {
std::cout << a << " ";
}
std::cout << std::endl;
return 0;
}
```
输出结果为:
```
6 8 10
```
其中,`std::transform()`算法可以将两个容器的元素一一对应进行操作,并将结果存储到第三个容器中。这里的lambda表达式`[](int a, int b) { return a + b + 1; }`表示对于两个输入的整数a和b,将它们相加并加上1,返回一个新的整数作为结果。最后,使用范围for循环遍历第三个容器z,输出结果。
python for循环中遍历两个不同长度的列表
可以使用zip()函数将两个列表打包成元组,再用for循环进行遍历。如果两个列表长度不同,则循环会以最短的列表长度为基准。代码示例如下:
```
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c', 'd']
for x, y in zip(list1, list2):
print(x, y)
```
输出结果为:
```
1 a
2 b
3 c
```
相关推荐
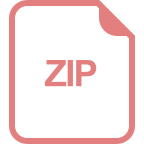
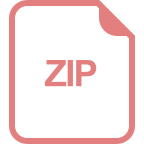
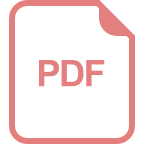












