pyqt如何取消鼠标右键
时间: 2023-10-24 19:10:25 浏览: 163
可以通过重写 QWidget 的 mousePressEvent 和 contextMenuEvent 方法来实现取消鼠标右键的功能。
以下是一个例子:
```python
from PyQt5.QtWidgets import QWidget, QApplication
class MyWidget(QWidget):
def __init__(self):
super().__init__()
def mousePressEvent(self, event):
if event.button() == Qt.RightButton:
event.ignore()
def contextMenuEvent(self, event):
event.ignore()
if __name__ == '__main__':
app = QApplication([])
widget = MyWidget()
widget.show()
app.exec_()
```
在这个例子中,重写了 mousePressEvent 和 contextMenuEvent 方法,并在右键按下事件和上下文菜单事件中调用了 event.ignore() 方法,这样就可以取消鼠标右键的功能。
相关问题
pyqt 重写鼠标右键事件
可以通过重写 QWidget 的 contextMenuEvent() 函数来实现 PyQt 中鼠标右键事件的重写。具体实现代码如下:
```python
class MyWidget(QWidget):
def __init__(self):
super().__init__()
def contextMenuEvent(self, event):
menu = QMenu(self)
menu.addAction("Action 1")
menu.addAction("Action 2")
menu.exec_(event.globalPos())
```
在这个例子中,我们创建了一个自定义的 QWidget 类 MyWidget,并重写了它的 contextMenuEvent() 函数。在这个函数中,我们创建了一个 QMenu 对象,并添加了两个 QAction。最后,我们通过调用 QMenu 的 exec_() 函数来显示右键菜单。
使用pyqt实现鼠标右键拖拽图片平移
以下是使用PyQt实现鼠标右键拖拽图片平移的简单示例代码:
```python
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QApplication, QGraphicsScene, QGraphicsView
class ImageViewer(QGraphicsView):
def __init__(self, parent=None):
super(ImageViewer, self).__init__(parent)
# 创建场景
self.scene = QGraphicsScene(self)
self.setScene(self.scene)
# 加载图像
self.image = QPixmap('image.png')
# 添加图像到场景
self.image_item = self.scene.addPixmap(self.image)
# 开启拖拽模式
self.setDragMode(QGraphicsView.ScrollHandDrag)
# 重写鼠标按下事件
self.mousePressEvent = self.on_mouse_press_event
# 重写鼠标移动事件
self.mouseMoveEvent = self.on_mouse_move_event
def on_mouse_press_event(self, event):
# 如果是鼠标右键按下,则记录鼠标位置
if event.button() == Qt.RightButton:
self.last_mouse_pos = event.pos()
else:
super().mousePressEvent(event)
def on_mouse_move_event(self, event):
# 如果是鼠标右键移动,则计算偏移量并移动图像
if event.buttons() == Qt.RightButton:
offset = event.pos() - self.last_mouse_pos
self.image_item.moveBy(offset.x(), offset.y())
self.last_mouse_pos = event.pos()
else:
super().mouseMoveEvent(event)
if __name__ == '__main__':
app = QApplication([])
viewer = ImageViewer()
viewer.show()
app.exec_()
```
在这个示例中,我们重写了鼠标按下和鼠标移动事件。如果用户按下鼠标右键,则记录鼠标位置。在移动鼠标时,如果鼠标右键处于按下状态,则计算偏移量并移动图像。最后,我们将所有其他鼠标事件委托给父类处理。
阅读全文
相关推荐
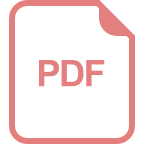



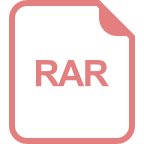








