c语言从一个文件夹中读取数据,处理结束后同名保存在另一个文件夹下
时间: 2023-07-31 08:05:27 浏览: 49
您可以使用C语言中的标准文件操作函数来完成这个任务。首先,您需要使用fopen()函数打开要读取的文件,并使用fread()函数读取文件中的数据。然后,您可以将读取的数据处理,执行您的任务,并将结果写入一个新文件。使用fclose()函数关闭文件以确保数据写入文件。最后,您可以使用fopen()函数重新打开目标文件,并使用fwrite()函数将处理后的数据写入该文件。
以下是处理程序框架的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define BUFFER_SIZE 256
int main() {
FILE *input_file, *output_file;
char buffer[BUFFER_SIZE];
size_t read_size;
// 打开源文件并读取数据
input_file = fopen("folder1/input.txt", "rb");
if (!input_file) {
printf("Failed to open input file\n");
exit(1);
}
read_size = fread(buffer, 1, BUFFER_SIZE, input_file);
fclose(input_file);
// 数据处理
// 将结果写入目标文件
output_file = fopen("folder2/output.txt", "wb");
if (!output_file) {
printf("Failed to open output file\n");
exit(1);
}
fwrite(buffer, 1, read_size, output_file);
fclose(output_file);
return 0;
}
```
请注意,在示例代码中,我们使用二进制模式打开文件。如果您的文件包含文本内容,您应该使用文本模式打开文件。
相关推荐
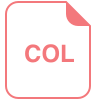













