用python实现模拟红绿灯
时间: 2023-07-12 22:15:36 浏览: 278
好的,我可以帮你实现模拟红绿灯的程序。以下是示例代码:
```python
import time
def red_light():
print("Red Light")
time.sleep(5) # 红灯持续5秒
def yellow_light():
print("Yellow Light")
time.sleep(2) # 黄灯持续2秒
def green_light():
print("Green Light")
time.sleep(5) # 绿灯持续5秒
while True:
red_light()
yellow_light()
green_light()
yellow_light()
```
在这个程序中,我们定义了三个函数分别代表红灯、黄灯和绿灯。在主循环中,我们按照红灯、黄灯、绿灯、黄灯的顺序循环执行这三个函数,模拟红绿灯的变化。
你可以根据需要调整红、黄、绿灯持续时间。同时,你也可以根据需要加入其他功能,比如在控制红绿灯的同时检测车流量等。
希望这个示例程序能对你有所帮助!
相关问题
python用程序模拟红绿灯
好的,可以通过使用Python中的time模块和循环语句来模拟红绿灯的过程。具体实现方式如下:
```python
import time
while True:
# 红灯
print("红灯")
time.sleep(5) # 延时5秒
# 绿灯
print("绿灯")
time.sleep(5) # 延时5秒
```
这个程序会循环打印“红灯”和“绿灯”并且每次会延时5秒钟。这样就可以通过程序模拟出红绿灯的过程了。当然,具体的实现方式可以根据需要进行调整。
python红绿灯控制系统
Python红绿灯控制系统是一种基于Python编程语言开发的交通信号灯控制系统。该系统可以用于模拟城市交通路口的交通信号灯控制,以实现更加高效的道路交通。
在该系统中,可以通过Python编程语言来控制交通信号灯的变换。通常,该系统中会使用GPIO控制模块,将GPIO输出接到对应的LED或其他指示灯上,以实现交通信号灯的控制。同时,该系统还可以通过传感器等设备来实现自动控制。
如果您想更深入了解Python红绿灯控制系统,可以学习相关的Python编程知识和GPIO控制技术,并参考一些开源的项目实例。
相关推荐
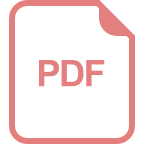
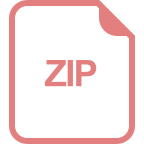












