多表联查怎么用java实现
时间: 2023-09-10 14:05:35 浏览: 81
多表联查可以通过使用Java的JDBC连接数据库,并使用SQL语句进行查询实现。
以下是一个示例代码:
```java
import java.sql.*;
public class MultiTableJoinExample {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost:3306/mydatabase";
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try{
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL,USER,PASS);
stmt = conn.createStatement();
String sql = "SELECT customers.name, orders.order_date, order_items.product_name " +
"FROM customers " +
"INNER JOIN orders ON customers.customer_id = orders.customer_id " +
"INNER JOIN order_items ON orders.order_id = order_items.order_id";
ResultSet rs = stmt.executeQuery(sql);
while(rs.next()){
String name = rs.getString("name");
String orderDate = rs.getString("order_date");
String productName = rs.getString("product_name");
System.out.print("Name: " + name);
System.out.print(", Order Date: " + orderDate);
System.out.println(", Product Name: " + productName);
}
rs.close();
stmt.close();
conn.close();
}catch(SQLException se){
se.printStackTrace();
}catch(Exception e){
e.printStackTrace();
}finally{
try{
if(stmt!=null)
stmt.close();
}catch(SQLException se2){
}
try{
if(conn!=null)
conn.close();
}catch(SQLException se){
se.printStackTrace();
}
}
}
}
```
在上述代码中,我们使用了JDBC驱动程序来连接MySQL数据库,并执行了一个SQL查询,该查询使用了多个表的联合查询。该查询返回了客户名称、订单日期和产品名称。然后,我们使用ResultSet对象从查询结果中获取数据,并在控制台上输出查询结果。最后,我们关闭了Connection、Statement和ResultSet对象,以释放资源。
请注意,在实际应用中,您需要根据自己的环境和需求进行修改和定制化。
阅读全文
相关推荐


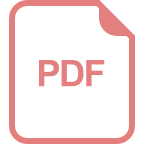












