编写c语言程序,文本文件student.txt中存放了若干名学生的学号和出勤次数,从文件中读取这些信息分别存档在两个不同的数组中,然后依次点名,根据出勤情况修改相应学生的出勤次数,最后将更新后的出勤情况再次写入文件student.txt
时间: 2023-12-27 12:04:33 浏览: 117
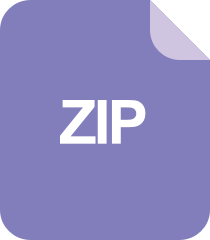
一个用C语言编写的学生信息管理系统,学生数据保存在文本文件中
下面是一个示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENTS 100
int read_students(const char *filename, int *ids, int *attendances) {
FILE *fp;
int id, attendance, count = 0;
fp = fopen(filename, "r");
if (fp == NULL) {
printf("Error opening file %s\n", filename);
return -1;
}
while (fscanf(fp, "%d %d", &id, &attendance) == 2 && count < MAX_STUDENTS) {
ids[count] = id;
attendances[count] = attendance;
count++;
}
fclose(fp);
return count;
}
void write_students(const char *filename, int *ids, int *attendances, int count) {
FILE *fp;
int i;
fp = fopen(filename, "w");
if (fp == NULL) {
printf("Error opening file %s\n", filename);
return;
}
for (i = 0; i < count; i++) {
fprintf(fp, "%d %d\n", ids[i], attendances[i]);
}
fclose(fp);
}
int main() {
int ids[MAX_STUDENTS], attendances[MAX_STUDENTS];
int count, i, id, attendance;
count = read_students("student.txt", ids, attendances);
if (count == -1) {
return 1;
}
for (i = 0; i < count; i++) {
printf("Student %d, attendance %d\n", ids[i], attendances[i]);
}
printf("\n");
for (i = 0; i < count; i++) {
printf("Call student %d (y/n): ", ids[i]);
if (scanf("%c", &c) != 1) {
printf("Error reading input\n");
return 1;
}
if (c == 'y' || c == 'Y') {
attendances[i]++;
}
}
write_students("student.txt", ids, attendances, count);
return 0;
}
```
这个程序首先定义了一个常量 `MAX_STUDENTS`,用于限制最大的学生数。然后定义了两个数组 `ids` 和 `attendances`,用于存放学号和出勤次数。接着定义了两个函数 `read_students` 和 `write_students`,分别用于从文件中读取学生信息和将更新后的信息写入文件。
在 `main` 函数中,首先调用 `read_students` 函数从文件中读取学生信息,并将返回值保存在变量 `count` 中。如果读取失败,程序会直接退出。然后使用一个循环依次点名,根据用户输入修改学生的出勤次数。最后调用 `write_students` 函数将更新后的信息写入文件。
阅读全文
相关推荐
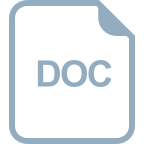

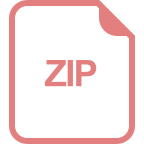
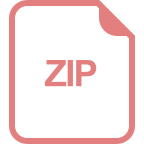
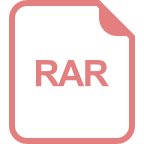
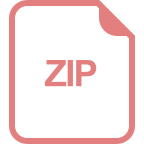
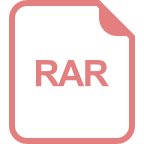
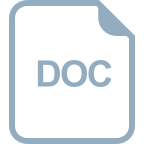
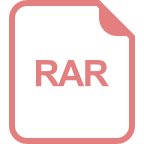
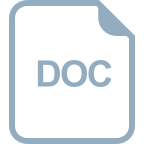
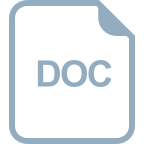
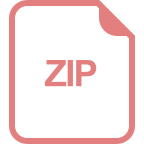
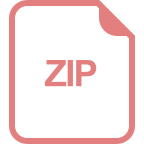
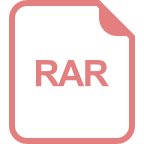
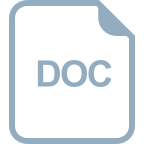
