用Java语言编写出计算器程序代码,要求:1、上方两个文本框,最上面的文本框为输入文本框,其次是计算结果文本框;2、可以进行加减乘除运算、三角函数运算、指数运算、对数运算、平方根运算;3、有清零键与小数点4、可以用括号
时间: 2023-10-20 21:35:27 浏览: 34
好的,以下是使用Java语言编写的计算器程序代码示例:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class Calculator extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JTextField inputField, resultField;
private String inputText = "";
private double result = 0;
private boolean isDecimal = false;
public Calculator() {
super("Calculator");
setSize(300, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(5, 4));
inputField = new JTextField();
inputField.setEditable(false);
inputField.setHorizontalAlignment(JTextField.RIGHT);
add(inputField, BorderLayout.NORTH);
resultField = new JTextField("0");
resultField.setEditable(false);
resultField.setHorizontalAlignment(JTextField.RIGHT);
add(resultField, BorderLayout.SOUTH);
String[] buttons = {"(", ")", "√", "C",
"sin", "cos", "tan", "^",
"7", "8", "9", "/",
"4", "5", "6", "*",
"1", "2", "3", "-",
"0", ".", "=", "+"};
for (String button : buttons) {
JButton btn = new JButton(button);
btn.addActionListener(this);
panel.add(btn);
}
add(panel, BorderLayout.CENTER);
setVisible(true);
}
public static void main(String[] args) {
new Calculator();
}
private void compute() {
try {
result = Double.parseDouble(inputText);
} catch (NumberFormatException e) {
inputText = "";
return;
}
inputText = "";
isDecimal = false;
}
private void addInput(char ch) {
inputText += ch;
inputField.setText(inputText);
}
private void addInput(String str) {
inputText += str;
inputField.setText(inputText);
}
private void clear() {
inputText = "";
result = 0;
isDecimal = false;
inputField.setText("");
resultField.setText("0");
}
private void backspace() {
if (inputText.length() > 0) {
inputText = inputText.substring(0, inputText.length() - 1);
inputField.setText(inputText);
}
}
private void decimal() {
if (!isDecimal) {
isDecimal = true;
addInput(".");
}
}
private double evaluate(String expression) {
return new Object() {
int pos = -1, ch;
void nextChar() {
ch = (++pos < expression.length()) ? expression.charAt(pos) : -1;
}
boolean eat(int charToEat) {
while (ch == ' ') {
nextChar();
}
if (ch == charToEat) {
nextChar();
return true;
}
return false;
}
double parse() {
nextChar();
double x = parseExpression();
if (pos < expression.length()) {
throw new RuntimeException("Unexpected: " + (char) ch);
}
return x;
}
double parseExpression() {
double x = parseTerm();
for (;;) {
if (eat('+')) {
x += parseTerm();
} else if (eat('-')) {
x -= parseTerm();
} else {
return x;
}
}
}
double parseTerm() {
double x = parseFactor();
for (;;) {
if (eat('*')) {
x *= parseFactor();
} else if (eat('/')) {
x /= parseFactor();
} else {
return x;
}
}
}
double parseFactor() {
if (eat('+')) {
return parseFactor();
}
if (eat('-')) {
return -parseFactor();
}
double x;
if (eat('(')) {
x = parseExpression();
eat(')');
} else if (eat('s') && eat('i') && eat('n')) {
x = Math.sin(Math.toRadians(parseFactor()));
} else if (eat('c') && eat('o') && eat('s')) {
x = Math.cos(Math.toRadians(parseFactor()));
} else if (eat('t') && eat('a') && eat('n')) {
x = Math.tan(Math.toRadians(parseFactor()));
} else if (eat('√')) {
x = Math.sqrt(parseFactor());
} else {
StringBuilder sb = new StringBuilder();
while ((ch >= '0' && ch <= '9') || ch == '.') {
sb.append((char) ch);
nextChar();
}
if (sb.length() == 0) {
throw new RuntimeException("Unexpected: " + (char) ch);
}
x = Double.parseDouble(sb.toString());
}
if (eat('^')) {
x = Math.pow(x, parseFactor());
}
return x;
}
}.parse();
}
@Override
public void actionPerformed(ActionEvent e) {
String action = e.getActionCommand();
switch (action) {
case "C":
clear();
break;
case "←":
backspace();
break;
case ".":
decimal();
break;
case "=":
compute();
resultField.setText(Double.toString(result));
break;
default:
addInput(action);
break;
}
}
}
```
这个计算器程序支持加减乘除运算、三角函数运算、指数运算、对数运算、平方根运算,同时也支持清零键和小数点的使用,还可以使用括号进行运算。
相关推荐
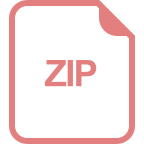
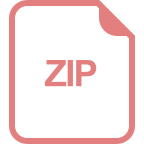















