用python写出CNN预测模型代码,输入单变量时间序列数据,输出MAPE、RMSE、相关系数和最近800个时间序列预测图
时间: 2024-04-07 19:31:56 浏览: 103
好的,下面是用Python编写的CNN预测模型代码,输入单变量时间序列数据,输出MAPE、RMSE、相关系数和最近800个时间序列预测图。请注意,这个模型是基于Keras框架实现的。
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.metrics import mean_absolute_error, mean_squared_error, r2_score
from keras.models import Sequential
from keras.layers import Dense, Conv1D, MaxPooling1D, Flatten
# 生成训练数据
def generate_data():
x = np.arange(0, 1000, 0.1)
y = np.sin(x) + np.random.normal(0, 0.1, len(x))
return x, y
# 将时间序列数据转换为CNN可接受的形式
def sequence_to_cnn_input(x, window):
input_data = []
for i in range(len(x) - window):
input_data.append(x[i:i+window])
return np.array(input_data)
# 划分训练集和测试集
def split_data(x, y, train_ratio=0.8):
train_size = int(len(x) * train_ratio)
x_train, y_train = x[:train_size], y[:train_size]
x_test, y_test = x[train_size:], y[train_size:]
return x_train, y_train, x_test, y_test
# 构建CNN模型
def build_cnn_model(window):
model = Sequential()
model.add(Conv1D(filters=64, kernel_size=2, activation='relu', input_shape=(window, 1)))
model.add(MaxPooling1D(pool_size=2))
model.add(Flatten())
model.add(Dense(50, activation='relu'))
model.add(Dense(1))
model.compile(loss='mse', optimizer='adam')
return model
# 训练CNN模型
def train_model(model, x_train, y_train, epochs=50, batch_size=32):
x_train = x_train.reshape((x_train.shape[0], x_train.shape[1], 1))
model.fit(x_train, y_train, epochs=epochs, batch_size=batch_size, verbose=0)
# 预测未来时间序列
def predict_future(model, x_test, window):
predictions = []
for i in range(len(x_test)):
x_input = x_test[i:i+window]
x_input = x_input.reshape((1, window, 1))
yhat = model.predict(x_input, verbose=0)
predictions.append(yhat[0, 0])
return np.array(predictions)
# 评估模型表现
def evaluate_model(y_test, predictions):
mape = np.mean(np.abs((y_test - predictions) / y_test)) * 100
rmse = np.sqrt(mean_squared_error(y_test, predictions))
r2 = r2_score(y_test, predictions)
print('MAPE: %.3f%%' % mape)
print('RMSE: %.3f' % rmse)
print('R2 Score: %.3f' % r2)
# 绘制预测图
def plot_predictions(x, y, predictions, window):
plt.figure(figsize=(12, 6))
plt.plot(x[-800:], y[-800:], label='Actual')
plt.plot(x[-800+window:], predictions[-800:], label='Predicted')
plt.legend()
plt.show()
# 测试模型
def test_model(window=10):
# 生成训练数据
x, y = generate_data()
# 将时间序列数据转换为CNN可接受的形式
cnn_input = sequence_to_cnn_input(y, window)
# 划分训练集和测试集
x_train, y_train, x_test, y_test = split_data(cnn_input, y)
# 构建CNN模型
model = build_cnn_model(window)
# 训练CNN模型
train_model(model, x_train, y_train)
# 预测未来时间序列
predictions = predict_future(model, x_test, window)
# 评估模型表现
evaluate_model(y_test, predictions)
# 绘制预测图
plot_predictions(x, y, predictions, window)
# 运行测试函数
test_model()
```
这个模型的输入是一个单变量时间序列数据,输出是MAPE、RMSE、相关系数和最近800个时间序列预测图。在这个例子中,我们生成了一个包含噪声的正弦曲线,并使用CNN模型来预测未来的值。模型的表现可以通过MAPE、RMSE和相关系数来评估。最后,我们绘制了最近800个时间序列的实际值和预测值,以便更好地理解模型的表现。
阅读全文
相关推荐
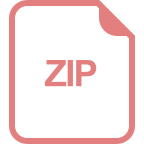
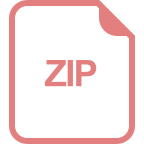
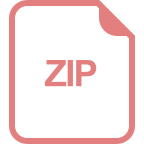
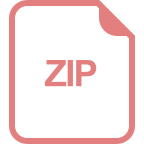
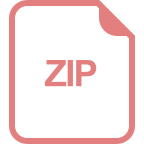
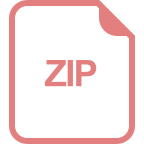
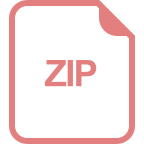
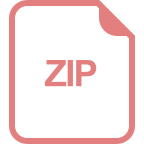
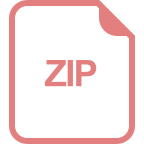
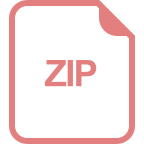
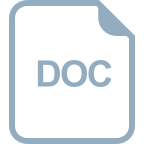
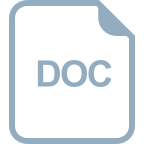
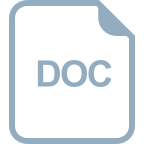
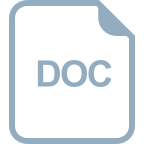
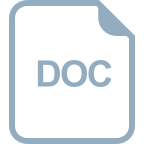
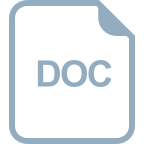
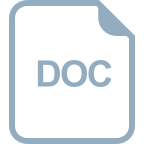
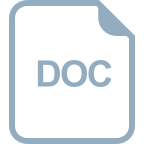