QT笔记之自定义窗口拖拽移动
时间: 2023-07-04 13:18:55 浏览: 82
好的,我可以为您讲解如何在QT中实现自定义窗口的拖拽移动。
首先,我们需要重写QWidget的mousePressEvent和mouseMoveEvent两个事件函数。
在mousePressEvent中,我们需要记录下鼠标按下时的坐标和窗口左上角的坐标,以便计算窗口移动的偏移量。
在mouseMoveEvent中,我们需要计算鼠标移动的偏移量,并将窗口的位置进行相应的调整。
以下是示例代码:
```cpp
void MyWindow::mousePressEvent(QMouseEvent *event)
{
if (event->button() == Qt::LeftButton)
{
m_dragPosition = event->globalPos() - frameGeometry().topLeft();
}
}
void MyWindow::mouseMoveEvent(QMouseEvent *event)
{
if (event->buttons() & Qt::LeftButton)
{
QPoint newPos = event->globalPos() - m_dragPosition;
move(newPos);
}
}
```
在上面的代码中,m_dragPosition是一个QPoint类型的成员变量,用于记录鼠标按下时的坐标和窗口左上角的坐标的差值。
最后,我们需要在窗口的构造函数中设置窗口的样式为Qt::FramelessWindowHint,以去掉窗口的边框和标题栏。
完整的示例代码如下:
```cpp
#include "mywindow.h"
#include <QMouseEvent>
MyWindow::MyWindow(QWidget *parent)
: QWidget(parent)
{
setFixedSize(400, 300);
setWindowFlags(Qt::FramelessWindowHint);
}
void MyWindow::mousePressEvent(QMouseEvent *event)
{
if (event->button() == Qt::LeftButton)
{
m_dragPosition = event->globalPos() - frameGeometry().topLeft();
}
}
void MyWindow::mouseMoveEvent(QMouseEvent *event)
{
if (event->buttons() & Qt::LeftButton)
{
QPoint newPos = event->globalPos() - m_dragPosition;
move(newPos);
}
}
```
希望这个简单的示例对您有所帮助。
相关推荐
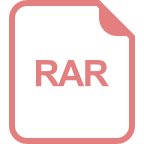














