python试用struct浮点数转十六进制
时间: 2023-08-25 16:27:52 浏览: 107
可以使用Python内置的`struct`模块将浮点数转换成二进制字节流,再用`binascii`模块将二进制流转换为十六进制字符串。具体实现如下:
```python
import struct
import binascii
x = 3.1415926
packed = struct.pack('f', x)
hex_float = binascii.hexlify(packed).decode('utf-8')
print(hex_float)
```
输出结果为:
```
f548f5c3
```
其中`struct.pack()`方法将浮点数`x`转换为4个字节的二进制字节流,`binascii.hexlify()`方法将二进制字节流转换为十六进制字符串。注意,`hexlify()`方法返回的是字节类型,需要使用`.decode('utf-8')`方法将其转换为字符串类型。
阅读全文
相关推荐
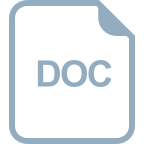
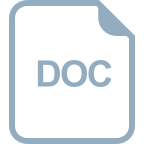
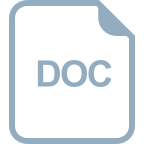
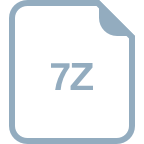





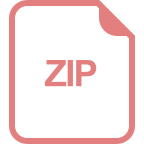
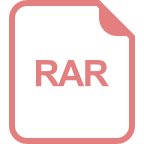
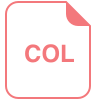
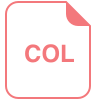
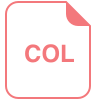
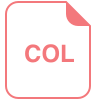


