实现一个计算机的功能,能够对两个实数实施加减乘除四则运算,选择一种功能性测试方法和结构性测试方法,对其进行测试
时间: 2025-01-05 18:40:59 浏览: 4
要实现一个计算机功能,用于进行两个实数的加减乘除四则运算,我们可以首先设计一个简单的程序,比如用Python编写一个函数:
```python
def arithmetic_operation(num1, num2, operation):
if operation == 'add':
return num1 + num2
elif operation == 'subtract':
return num1 - num2
elif operation == 'multiply':
return num1 * num2
elif operation == 'divide':
if num2 != 0:
return num1 / num2
else:
raise ValueError("Cannot divide by zero.")
else:
raise ValueError("Invalid operation.")
# 示例操作
result = arithmetic_operation(5, 3, 'add')
```
对于功能性的测试方法,我们应关注以下几个方面:
1. **边界条件**:测试最小值、最大值以及零等特殊输入,如极小实数、极大实数、浮点精度问题等。
2. **错误处理**:检查程序能否正确处理除数为零的情况,以及其他非法操作的异常情况。
3. **运算顺序**:对于除法,确认其左结合规则是否正确。
结构性测试方法包括:
1. **单元测试**:针对每个独立的函数(如`arithmetic_operation`),分别测试各个操作是否按照预期工作。
2. **集成测试**:测试整个系统(如用户界面或API)能否正确地组合和传递参数,并得到正确的结果。
3. **代码覆盖率**:确保测试用例覆盖了所有可能的代码路径,包括所有分支和循环。
阅读全文
相关推荐



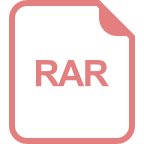














