C#不使用图像处理库实现图像模板匹配
时间: 2024-05-16 09:16:25 浏览: 11
实现图像模板匹配可以使用C#的System.Drawing命名空间中的Bitmap类操作图像。下面是一个简单的示例代码,它使用滑动窗口的方式在一张图像中搜索目标图像并找到最佳匹配位置。
```csharp
private Point FindTemplate(Bitmap source, Bitmap template)
{
int sourceWidth = source.Width;
int sourceHeight = source.Height;
int templateWidth = template.Width;
int templateHeight = template.Height;
double minMSE = double.MaxValue;
Point minPoint = Point.Empty;
// 遍历图像
for (int y = 0; y < sourceHeight - templateHeight; y++)
{
for (int x = 0; x < sourceWidth - templateWidth; x++)
{
double mse = 0;
// 计算均方误差MSE
for (int j = 0; j < templateHeight; j++)
{
for (int i = 0; i < templateWidth; i++)
{
Color sourceColor = source.GetPixel(x + i, y + j);
Color templateColor = template.GetPixel(i, j);
double diffR = sourceColor.R - templateColor.R;
double diffG = sourceColor.G - templateColor.G;
double diffB = sourceColor.B - templateColor.B;
mse += (diffR * diffR + diffG * diffG + diffB * diffB) / (templateWidth * templateHeight);
}
}
// 更新最小MSE值和坐标
if (mse < minMSE)
{
minMSE = mse;
minPoint = new Point(x, y);
}
}
}
return minPoint;
}
```
该方法的原理是遍历整个源图像,每次取出一个与目标图像大小相同的矩形区域,计算该区域内的均方误差MSE值。MSE值越小说明源图像与目标图像的匹配程度越高,因此每次记录下最小MSE值和对应的坐标。最后返回最佳匹配位置的坐标。
需要注意的是,该方法只能实现简单的图像模板匹配,对于复杂的情况可能会出现误差较大的情况。如果需要更高的匹配精度,可以考虑使用一些专业的图像处理库,如Emgu CV等。
相关推荐
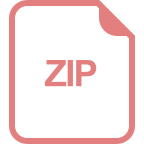
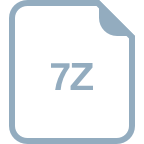
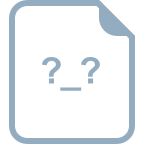














