人机交互界面设计代码
时间: 2023-07-02 10:21:09 浏览: 257
人机交互界面设计是一个较为复杂的过程,需要综合考虑用户需求、界面美观与易用性等多个方面。一般来说,人机交互界面设计包括前端和后端两个部分。
前端部分主要涉及用户界面设计和交互设计,需要使用HTML、CSS、JavaScript等技术实现,以下是一个简单的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>人机交互界面示例</title>
<style>
/* 样式表,用于美化界面 */
body {
background-color: #f0f0f0;
font-family: Arial, sans-serif;
}
input[type="text"] {
width: 200px;
height: 30px;
border: 1px solid #ccc;
border-radius: 5px;
padding: 5px;
font-size: 16px;
}
button {
width: 80px;
height: 30px;
background-color: #0077cc;
color: #fff;
border: none;
border-radius: 5px;
font-size: 16px;
}
</style>
<script>
// JavaScript代码,用于实现交互功能
function submitForm() {
// 获取输入框的值
var input = document.getElementById("inputText").value;
// 向后端发送请求
// ...
// 更新界面
document.getElementById("result").innerHTML = "您输入的内容是:" + input;
}
</script>
</head>
<body>
<h1>人机交互界面示例</h1>
<p>请输入内容:</p>
<input type="text" id="inputText">
<button onclick="submitForm()">提交</button>
<br>
<br>
<div id="result"></div>
</body>
</html>
```
后端部分主要涉及数据处理和业务逻辑,需要使用后端编程语言(如Java、Python等)实现,以下是一个简单的示例代码:
```java
import javax.servlet.http.*;
import javax.servlet.*;
import java.io.*;
public class HelloWorld extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 获取前端传递的参数
String input = request.getParameter("input");
// 处理业务逻辑
// ...
// 返回结果
response.setContentType("text/html;charset=utf8");
PrintWriter out = response.getWriter();
out.println("<html>");
out.println("<head><title>Hello World</title></head>");
out.println("<body>");
out.println("<p>您输入的内容是:" + input + "</p>");
out.println("</body>");
out.println("</html>");
out.close();
}
}
```
总之,人机交互界面设计需要综合考虑多个方面,包括用户需求、界面美观与易用性等。在实现过程中,需要使用前端和后端技术实现界面和交互功能,并进行数据处理和业务逻辑实现。
阅读全文
相关推荐
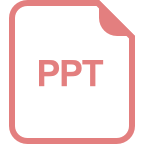
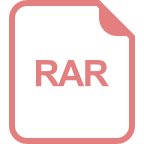
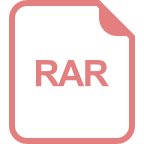
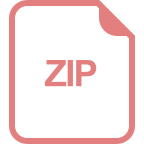
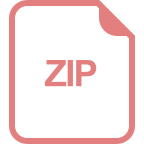
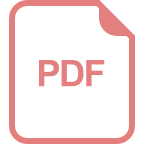
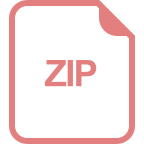
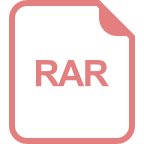
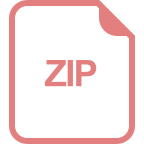
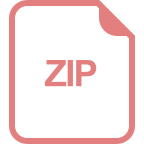
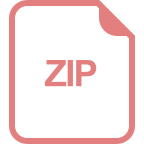
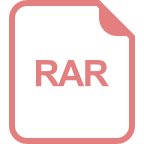
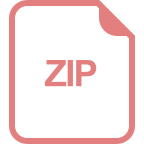
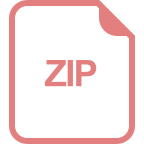
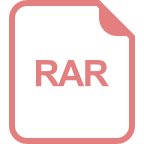
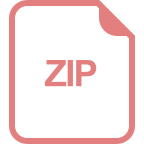
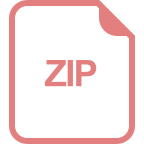