LocalDateTime类型 Json如何序列化时间戳
时间: 2023-07-28 18:37:48 浏览: 52
可以使用Jackson库将LocalDateTime类型转换为时间戳进行Json序列化。
首先,需要在pom.xml文件中添加Jackson的依赖:
```xml
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>${jackson.version}</version>
</dependency>
```
然后,在代码中使用ObjectMapper类进行序列化,可以通过调用configure方法设置序列化特性。使用writeValueAsString方法将Java对象序列化为Json字符串,例如:
```java
ObjectMapper mapper = new ObjectMapper();
mapper.configure(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS, true);
String json = mapper.writeValueAsString(localDateTime);
```
其中,WRITE_DATES_AS_TIMESTAMPS设置为true表示将日期格式化为时间戳。
注意:这种方式序列化的时间戳是以毫秒为单位的,如果需要以其他精度进行序列化,可以使用Jackson提供的其他特性。
相关问题
json的LocalDateTime 如何反序列化
根据提供的引用内容,我们可以得知在JSON中,时间通常以字符串的形式表示。而在Java中,我们可以使用LocalDateTime类来表示日期和时间。因此,我们需要将JSON中的时间字符串反序列化为LocalDateTime对象。
以下是将JSON中的时间字符串反序列化为LocalDateTime对象的示例代码:
```java
import com.fasterxml.jackson.databind.ObjectMapper;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class JsonUtil {
private static final ObjectMapper objectMapper = new ObjectMapper();
private static final DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd'T'HH:mm:ss.SSS");
public static LocalDateTime deserializeLocalDateTime(String json) throws Exception {
String dateStr = json.substring(json.indexOf("(") + 1, json.indexOf(")"));
LocalDateTime dateTime = LocalDateTime.parse(formatter.format(Long.parseLong(dateStr)), formatter);
return dateTime;
}
}
```
在上述代码中,我们使用了Jackson库的ObjectMapper类来反序列化JSON字符串。我们还使用了DateTimeFormatter类来指定日期时间格式。在deserializeLocalDateTime方法中,我们首先从JSON字符串中提取时间戳,然后将其转换为LocalDateTime对象。
LocalDateTime转化为秒的时间戳
要将LocalDateTime转换为秒的时间戳,可以使用以下方法:localDateTime.atZone(ZoneId.systemDefault()).toEpochSecond()。这个方法会将LocalDateTime转换为系统默认时区下的时间戳(秒)。需要注意的是,如果你的系统默认时区不是UTC+8时区,那么通过这种方式转换的时间戳可能会有误差。另外,需要注意的是LocalDateTime不能直接转换为Unix时间戳,因为它的toEpochSecond方法需要传入ZoneOffset参数。如果你希望得到一个可用于所有时区的时间戳,最好将LocalDateTime替换为Instant类型,因为Instant类型表示的是从标准的UTC时间1970年1月1日00:00:00开始的时间。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [LocalDateTime转换为时间戳(秒)](https://blog.csdn.net/huang007guo/article/details/129414291)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [将LocalDate和LocalDateTime序列化为Unix时间戳](https://blog.csdn.net/weixin_42299427/article/details/118799590)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
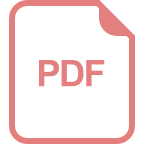












