现在有⼀个导出EXCEL表格数据的接⼝,因为数据量过⼤,导出时间过⻓,如何解决这个问题, 请写出思路和关键代码。
时间: 2023-06-12 16:03:23 浏览: 102
针对导出Excel表格数据时间过长的问题,可以考虑以下几种解决方案:
1. 分页导出数据:将数据分为多个页面,每次只导出一页数据,避免一次性导出过多数据导致时间过长。可以通过设置参数控制每页数据的数量。
2. 异步导出数据:将导出数据的操作放到后台异步处理,让用户可以继续使用系统,不必等待导出完成。可以使用Python的Celery来实现异步任务。
3. 数据压缩:对于数据量较大的情况,可以考虑将导出的数据进行压缩,减少导出时间和网络传输时间。
4. 前端优化:前端页面可以使用分页展示数据,避免一次性加载过多数据导致界面卡顿。同时可以使用loading动画提高用户体验。
关键代码如下:
1. 分页导出数据
```python
import xlwt
from django.core.paginator import Paginator
def export_data(request):
data = get_data() # 获取数据
page = request.GET.get('page', 1) # 获取当前页码
per_page = 100 # 设置每页数据量
paginator = Paginator(data, per_page)
page_data = paginator.page(page)
response = HttpResponse(content_type='application/ms-excel')
response['Content-Disposition'] = 'attachment; filename="data.xls"'
wb = xlwt.Workbook(encoding='utf-8')
ws = wb.add_sheet('Sheet1')
row_num = 0
columns = ['字段1', '字段2', '字段3', '字段4'] # 设置表头
for col_num, column_title in enumerate(columns):
ws.write(row_num, col_num, column_title)
for row in page_data:
row_num += 1
row = [row.field1, row.field2, row.field3, row.field4] # 获取每一行数据
for col_num, cell_value in enumerate(row):
ws.write(row_num, col_num, cell_value)
wb.save(response)
return response
```
2. 异步导出数据
```python
import xlwt
from celery import shared_task
@shared_task
def export_data_task():
data = get_data() # 获取数据
response = HttpResponse(content_type='application/ms-excel')
response['Content-Disposition'] = 'attachment; filename="data.xls"'
wb = xlwt.Workbook(encoding='utf-8')
ws = wb.add_sheet('Sheet1')
row_num = 0
columns = ['字段1', '字段2', '字段3', '字段4'] # 设置表头
for col_num, column_title in enumerate(columns):
ws.write(row_num, col_num, column_title)
for row in data:
row_num += 1
row = [row.field1, row.field2, row.field3, row.field4] # 获取每一行数据
for col_num, cell_value in enumerate(row):
ws.write(row_num, col_num, cell_value)
wb.save(response)
return response
def export_data(request):
export_data_task.delay() # 异步执行导出任务
return HttpResponse('正在导出数据,请稍后查看下载文件')
```
3. 数据压缩
```python
import xlwt
import gzip
from django.views.decorators.gzip import gzip_page
@gzip_page
def export_data(request):
data = get_data() # 获取数据
response = HttpResponse(content_type='application/ms-excel')
response['Content-Disposition'] = 'attachment; filename="data.xls.gz"'
wb = xlwt.Workbook(encoding='utf-8')
ws = wb.add_sheet('Sheet1')
row_num = 0
columns = ['字段1', '字段2', '字段3', '字段4'] # 设置表头
for col_num, column_title in enumerate(columns):
ws.write(row_num, col_num, column_title)
for row in data:
row_num += 1
row = [row.field1, row.field2, row.field3, row.field4] # 获取每一行数据
for col_num, cell_value in enumerate(row):
ws.write(row_num, col_num, cell_value)
wb.save(response)
compressed_content = gzip.compress(response.content) # 压缩数据
response = HttpResponse(compressed_content, content_type='application/ms-excel')
response['Content-Encoding'] = 'gzip'
return response
```
4. 前端优化
前端页面使用分页展示数据,使用loading动画可以使用第三方库,如ElementUI等。
相关推荐


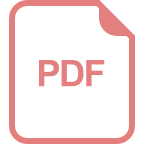
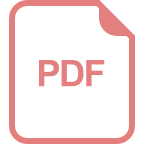
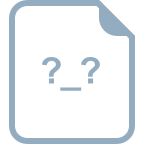
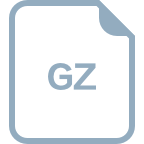
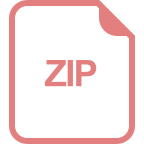