python结构光三维重建
时间: 2023-07-11 18:57:34 浏览: 193
Python可以使用OpenCV和NumPy等开源库进行结构光三维重建。以下是一个简单的示例代码:
```python
import cv2
import numpy as np
# 加载结构光图像
pattern = cv2.imread('pattern.png', cv2.IMREAD_GRAYSCALE)
image1 = cv2.imread('image1.png', cv2.IMREAD_GRAYSCALE)
image2 = cv2.imread('image2.png', cv2.IMREAD_GRAYSCALE)
# 计算相位差
phase = np.arctan2(np.sin(image1 - image2), np.cos(image1 - image2))
# 计算深度信息
h, w = pattern.shape
scale = 8
depth = phase * scale / (2 * np.pi)
depth = cv2.resize(depth, (w, h))
# 显示深度图像
cv2.imshow('depth', depth)
cv2.waitKey(0)
# 三维重建
fx = fy = 1000
cx = w / 2
cy = h / 2
points = []
for y in range(h):
for x in range(w):
z = depth[y, x]
if z != 0:
points.append((x, y, z))
points = np.array(points)
points[:, 0] = (points[:, 0] - cx) * points[:, 2] / fx
points[:, 1] = (points[:, 1] - cy) * points[:, 2] / fy
# 将点云保存为PLY文件
with open('pointcloud.ply', 'w') as f:
f.write('ply\n')
f.write('format ascii 1.0\n')
f.write('element vertex %d\n' % len(points))
f.write('property float x\n')
f.write('property float y\n')
f.write('property float z\n')
f.write('end_header\n')
for point in points:
f.write('%f %f %f\n' % tuple(point))
```
注:以上代码仅为示例,实际应用中需要根据具体情况进行修改和优化。
阅读全文
相关推荐
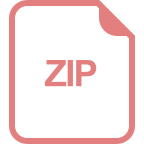



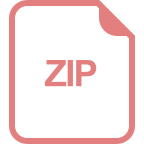
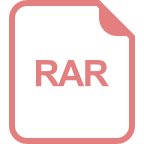
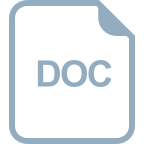
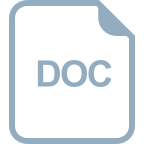







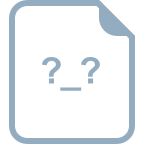