antd的select二级联动
时间: 2023-06-24 16:08:28 浏览: 62
antd的Select组件可以通过设置`onSelect`事件和动态改变`options`属性来实现二级联动。
假设我们有以下数据:
```js
const options = [
{
label: '北京',
value: 'bj',
children: [
{
label: '海淀区',
value: 'hd',
},
{
label: '朝阳区',
value: 'cy',
},
],
},
{
label: '上海',
value: 'sh',
children: [
{
label: '浦东新区',
value: 'pd',
},
{
label: '徐汇区',
value: 'xh',
},
],
},
];
```
我们需要在Select组件中,选中第一级选项后,动态的更新第二级选项。实现的代码如下:
```jsx
import React, { useState } from 'react';
import { Select } from 'antd';
const { Option } = Select;
const options = [
{
label: '北京',
value: 'bj',
children: [
{
label: '海淀区',
value: 'hd',
},
{
label: '朝阳区',
value: 'cy',
},
],
},
{
label: '上海',
value: 'sh',
children: [
{
label: '浦东新区',
value: 'pd',
},
{
label: '徐汇区',
value: 'xh',
},
],
},
];
function CascadingSelect() {
const [cities, setCities] = useState(options[0].children);
const handleCityChange = (value) => {
const city = options.find((item) => item.value === value);
setCities(city.children);
};
return (
<div>
<Select defaultValue={options[0].value} style={{ width: 120 }} onSelect={handleCityChange}>
{options.map((option) => (
<Option key={option.value} value={option.value}>
{option.label}
</Option>
))}
</Select>
<Select style={{ width: 120 }} defaultValue={cities[0].value}>
{cities.map((city) => (
<Option key={city.value} value={city.value}>
{city.label}
</Option>
))}
</Select>
</div>
);
}
export default CascadingSelect;
```
在这个例子中,我们在第一个Select组件中设置了`onSelect`事件,当选中北京或上海时,会触发`handleCityChange`函数,动态的更新第二个Select组件中的选项。
相关推荐
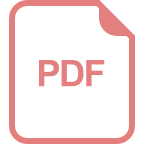
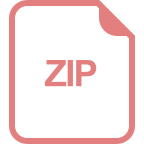
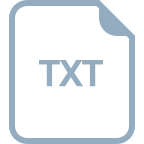














