如何在javaFX中写代码,实现一个静态的时间钟表
时间: 2024-12-06 16:24:33 浏览: 15
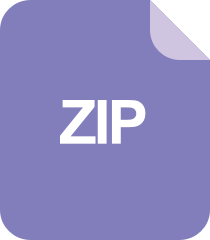
JavaFX实现一个画板
在JavaFX中创建一个静态时间钟通常会涉及到`javafx.scene.layout.StackPane`、`javafx.scene.control.Label`和`javafx.animation.AnimationTimer`。以下是一个简单的示例,展示如何创建一个基本的静态数字钟:
```java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.scene.text.Font;
import javafx.stage.Stage;
import javafx.util.Duration;
public class ClockApp extends Application {
private Label clockLabel;
private AnimationTimer timer;
@Override
public void start(Stage primaryStage) {
// 设置舞台背景和尺寸
primaryStage.setTitle("JavaFX 静态时间钟");
primaryStage.setScene(new Scene(new StackPane(), 300, 150));
primaryStage.show();
// 创建并初始化时间标签
clockLabel = new Label();
clockLabel.setFont(Font.font(48)); // 设置字体大小
clockLabel.setText("00:00:00"); // 初始显示时间为00:00:00
clockLabel.setStyle("-fx-text-fill: white;"); // 设置文字颜色
// 添加到场景
StackPane root = (StackPane) primaryStage.getScene().getRoot();
root.getChildren().add(clockLabel);
// 初始化定时器,每秒更新一次时间
timer = new AnimationTimer() {
@Override
public void handle(long now) {
updateClockTime();
}
};
timer.start(); // 开始计时
}
private void updateClockTime() {
// 更新时间到新的实例
String currentTime = getTimeString(System.currentTimeMillis());
clockLabel.setText(currentTimeString);
}
// 根据毫秒获取HH:mm:ss格式的时间字符串
private String getTimeString(long time) {
int hours = (int) ((time / (1000 * 60 * 60)) % 24);
int minutes = (int) ((time / (1000 * 60)) % 60);
int seconds = (int) ((time / 1000) % 60);
return String.format("%02d:%02d:%02d", hours, minutes, seconds);
}
public static void main(String[] args) {
launch(args);
}
}
阅读全文
相关推荐
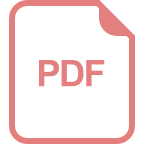
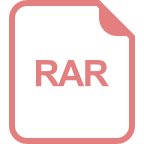
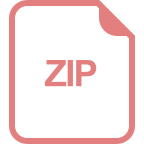
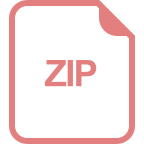
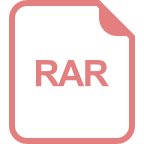
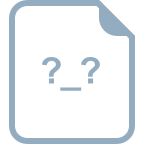
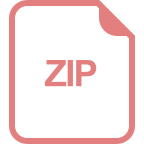
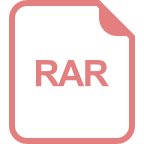
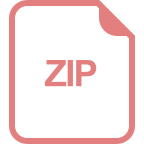
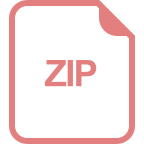
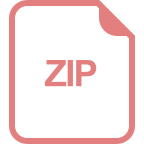
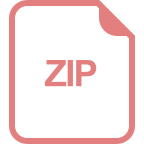