esp8266采集温湿度数据,然后上传到mysql数据库中
时间: 2023-06-17 13:04:50 浏览: 167
要将esp8266采集到的温湿度数据上传到MySQL数据库中,可以按照以下步骤进行:
1. 连接esp8266和传感器:使用esp8266连接温湿度传感器,采集数据。
2. 连接WiFi网络:使用esp8266连接WiFi网络,确保可以访问互联网。
3. 连接MySQL数据库:在互联网上创建一个MySQL数据库,并使用esp8266连接到该数据库。
4. 创建数据表:在MySQL数据库中创建一个数据表,用于存储温湿度数据。
5. 编写代码:使用Arduino IDE编写代码,实现esp8266采集温湿度数据并上传到MySQL数据库中。
下面是一个简单的示例代码:
```c++
#include <ESP8266WiFi.h>
#include <MySQL_Connection.h>
#include <MySQL_Cursor.h>
#include <DHT.h>
#define DHTPIN D4 // 温湿度传感器连接的引脚
#define DHTTYPE DHT11 // 温湿度传感器类型
#define WIFI_SSID "your_ssid" // WiFi网络名称
#define WIFI_PASSWORD "your_password" // WiFi网络密码
#define MYSQL_HOST "your_mysql_host" // MySQL数据库主机地址
#define MYSQL_PORT 3306 // MySQL数据库端口号
#define MYSQL_USER "your_mysql_user" // MySQL数据库用户名
#define MYSQL_PASSWORD "your_mysql_password" // MySQL数据库密码
#define MYSQL_DATABASE "your_mysql_database" // MySQL数据库名称
DHT dht(DHTPIN, DHTTYPE);
WiFiClient client;
MySQL_Connection conn((Client *)&client);
void setup() {
Serial.begin(9600);
delay(100);
// 连接WiFi网络
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
// 连接MySQL数据库
Serial.println("Connecting to MySQL database...");
if (conn.connect(MYSQL_HOST, MYSQL_PORT, MYSQL_USER, MYSQL_PASSWORD)) {
Serial.println("Connected to MySQL database!");
} else {
Serial.println("Failed to connect to MySQL database.");
while (1);
}
// 创建数据表
Serial.println("Creating table...");
MySQL_Cursor *cursor = new MySQL_Cursor(&conn);
cursor->execute("CREATE TABLE IF NOT EXISTS temperature_humidity (id INT UNSIGNED AUTO_INCREMENT PRIMARY KEY, temperature FLOAT, humidity FLOAT)");
delete cursor;
// 初始化温湿度传感器
dht.begin();
}
void loop() {
// 采集温湿度数据
float temperature = dht.readTemperature();
float humidity = dht.readHumidity();
// 将数据上传到MySQL数据库中
if (conn.connected()) {
Serial.println("Inserting data into MySQL database...");
char query[128];
sprintf(query, "INSERT INTO temperature_humidity (temperature, humidity) VALUES (%f, %f)", temperature, humidity);
MySQL_Cursor *cursor = new MySQL_Cursor(&conn);
cursor->execute(query);
delete cursor;
Serial.println("Data inserted into MySQL database!");
} else {
Serial.println("Failed to insert data into MySQL database.");
}
delay(10000); // 每10秒钟采集一次数据
}
```
在这个示例中,我们使用了ESP8266WiFi、MySQL_Connection和MySQL_Cursor库来连接WiFi网络和MySQL数据库,并且使用DHT库来读取温湿度传感器的数据。在setup()函数中,我们连接WiFi网络和MySQL数据库,并创建一个名为“temperature_humidity”的数据表。在loop()函数中,我们采集温湿度数据,并将其插入到MySQL数据库中。
请确保在使用该示例代码前,已经将所有的配置信息(如WiFi网络名称、密码、MySQL数据库主机地址、用户名、密码和数据库名称)替换为自己的信息。
相关推荐
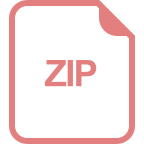
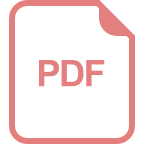














