密码包含大小写字母转为纯大写
时间: 2023-11-18 07:27:43 浏览: 29
可以使用字符串的 `toupper()` 函数将密码中的所有字母转换为大写字母。
以下是一个示例代码,可以将输入的字符串密码转换为纯大写字母的密码:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main()
{
string password;
cout << "Please enter the password: ";
getline(cin, password);
// 将密码中的所有字母转换为大写字母
for (int i = 0; i < password.length(); i++)
{
password[i] = toupper(password[i]);
}
cout << "The converted password is: " << password;
return 0;
}
```
输入示例:
```
Please enter the password: AbCdEfG123
```
输出示例:
```
The converted password is: ABCDEFG123
```
相关问题
输入任意包含大小写字母的字符串,将其中的大小写字母转换为小写,并将原小写字母转为大写不用内置函数
好的,以下是 Python 代码实现:
```python
s = input("请输入字符串:")
new_s = ""
for c in s:
if c.islower():
new_s += c.upper()
elif c.isupper():
new_s += c.lower()
else:
new_s += c
print(new_s)
```
以上代码中,首先通过 `input()` 函数获取用户输入的字符串,并创建一个空字符串 `new_s` 用于存储转换后的字符串。然后使用 `for` 循环遍历输入字符串中的每一个字符,如果该字符是小写字母,则将其转换为大写并添加到 `new_s` 中;如果该字符是大写字母,则将其转换为小写并添加到 `new_s` 中;否则直接将该字符添加到 `new_s` 中。最后输出转换后的字符串。
输入小写英文字母,把字母转为大写输出,并且输出字母大小写对应的ascll码值
这段代码的功能是输入小写英文字母,将其转换为大写字母并输出,同时输出转换后的字母对应的ASCII码值。具体实现过程如下:
1. 首先要引入stdio.h库。
2. 使用scanf_s函数读入一个小写英文字母。
3. 判断输入的字母是否在a~p的范围内,如果不是则提示输入有误并重新读入。
4. 将小写字母转换为大写字母,转换方法是将其ASCII码值减去32。
5. 输出转换后的大写字母和其对应的ASCII码值。
下面是代码示例:
```
#include <stdio.h>
int main(void) {
char ch; // 输入的小写英文字母
char ch1; // 转换后的大写英文字母
printf("请输入一个小写英文字母:\n");
scanf_s("%c", &ch);
getchar(); // 将缓冲区的回车符读入,避免在后面作为有效字符读入
while (ch < 97 || ch > 112) {
printf("您的输入有误,请重新输入:");
scanf_s("%c", &ch);
getchar();
}
ch1 = ch - 32; // 小写转换为大写
printf("转换后的大写字母为:%c\n该字母的ASCII码为:%d", ch1, ch1);
return 0;
}
```
输入小写字母后,程序会将其转换为大写字母并输出,同时输出转换后的字母对应的ASCII码值。
相关推荐
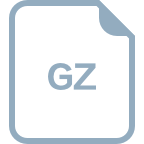
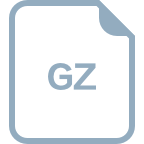












