RegGAN加入attention代码
时间: 2023-07-02 12:09:36 浏览: 127
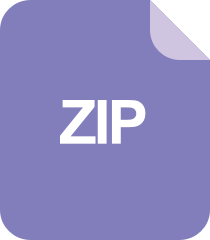
attention代码
好的,以下是一个简单的示例,展示如何将attention机制加入到RegGAN中:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class RegGAN(nn.Module):
def __init__(self, input_size, output_size, hidden_size, num_layers, dropout, attention_size):
super(RegGAN, self).__init__()
# 定义生成器
self.generator = nn.Sequential(
nn.Linear(input_size, hidden_size),
nn.LeakyReLU(0.2),
nn.Dropout(dropout),
nn.Linear(hidden_size, hidden_size),
nn.LeakyReLU(0.2),
nn.Dropout(dropout),
nn.Linear(hidden_size, output_size),
nn.Tanh()
)
# 定义鉴别器
self.discriminator = nn.Sequential(
nn.Linear(output_size, hidden_size),
nn.LeakyReLU(0.2),
nn.Linear(hidden_size, hidden_size),
nn.LeakyReLU(0.2),
nn.Linear(hidden_size, 1),
nn.Sigmoid()
)
# 定义attention机制
self.attention = nn.Sequential(
nn.Linear(hidden_size, attention_size),
nn.Tanh(),
nn.Linear(attention_size, 1),
nn.Softmax(dim=1)
)
def forward(self, x):
# 生成器前向传播
x_gen = self.generator(x)
# 鉴别器前向传播
d_out = self.discriminator(x_gen)
# 计算attention权重
attn_weights = self.attention(x_gen)
# 将attention权重与生成器输出相乘
x_out = (attn_weights * x_gen).sum(dim=1)
return x_out, d_out
```
在上面的代码中,我们添加了一个名为`attention`的新神经网络层,它接受生成器的输出作为输入,并输出一个权重向量。我们使用一个简单的两层全连接神经网络来实现attention机制,其中第一层将生成器输出映射到一个较小的维度,第二层将其映射到一个标量。最后,我们使用softmax函数将这个标量转换为一个权重向量,该向量的每个元素表示相应生成器输出的相对重要性。
然后,在`forward`函数中,我们首先通过生成器生成一批数据,然后将其传递给鉴别器。接下来,我们将生成器的输出传递给attention层,获取attention权重。最后,我们将attention权重与生成器的输出相乘,并对结果进行求和,以得到最终的输出。
阅读全文
相关推荐
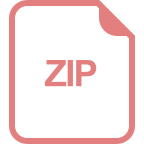
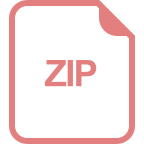
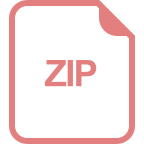
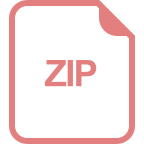
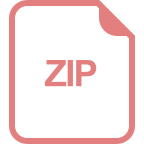