DPN网络模型结构详解
时间: 2023-11-17 22:49:05 浏览: 181
DPN(Dual Path Network)网络模型是一种深度神经网络,由微软亚洲研究院提出。它的设计思想是通过引入双通道和交叉连接来增强网络的表达能力,提高模型的准确性和稳定性。
DPN网络模型的主要特点是双通道和交叉连接。双通道是指网络中同时存在两个并行的路径,每个路径都有自己的卷积和池化操作。交叉连接是指两个并行路径中的某些层通过连接的方式进行交叉,实现信息的共享和流动。
DPN网络模型可以分为两个阶段:前半部分是特征提取阶段,后半部分是分类阶段。特征提取阶段由多个双通道和交叉连接的DPN模块构成,每个DPN模块包含两个双通道和一个交叉连接。分类阶段由全局平均池化层和全连接层组成。
DPN网络模型的优点在于它可以同时利用两个通道的信息,增强了网络的表达能力。交叉连接可以促进信息的共享和流动,进一步提高了模型的准确性和稳定性。此外,DPN网络模型还采用了批标准化、残差连接等技术,进一步提高了网络的性能。
总的来说,DPN网络模型是一种非常有效的深度神经网络,它的双通道和交叉连接设计思想为图像分类、目标检测等任务的解决提供了一种新的思路。
相关问题
利用pytorch框架设计基于DPN92模型的图像检测与分类输出坐标、大小和种类
首先,我们需要安装PyTorch和其他必要的库。可以使用以下命令安装PyTorch:
```
pip install torch torchvision
```
其他库可以使用以下命令安装:
```
pip install numpy pandas matplotlib opencv-python
```
接下来,我们需要下载DPN92预训练模型的权重。可以使用以下命令下载:
```
wget https://github.com/c0nn3r/DPN/releases/download/v2.0/DPN92_extra_5k.pth.tar
```
现在开始设计模型。我们将使用PyTorch中的预训练模型和自定义头来实现图像检测和分类。以下是完整的代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
import torchvision.transforms as transforms
import cv2
import numpy as np
# Define the custom head for object detection
class DetectionHead(nn.Module):
def __init__(self, in_channels, num_classes):
super(DetectionHead, self).__init__()
self.conv1 = nn.Conv2d(in_channels, in_channels, kernel_size=3, stride=1, padding=1)
self.bn1 = nn.BatchNorm2d(in_channels)
self.conv2 = nn.Conv2d(in_channels, in_channels, kernel_size=3, stride=1, padding=1)
self.bn2 = nn.BatchNorm2d(in_channels)
self.conv3 = nn.Conv2d(in_channels, in_channels, kernel_size=3, stride=1, padding=1)
self.bn3 = nn.BatchNorm2d(in_channels)
self.conv4 = nn.Conv2d(in_channels, num_classes * 5, kernel_size=3, stride=1, padding=1)
def forward(self, x):
x = F.relu(self.bn1(self.conv1(x)))
x = F.relu(self.bn2(self.conv2(x)))
x = F.relu(self.bn3(self.conv3(x)))
x = self.conv4(x)
return x
# Define the model
class DPN92Detection(nn.Module):
def __init__(self, num_classes):
super(DPN92Detection, self).__init__()
self.dpn92 = torch.hub.load('rwightman/pytorch-dpn-pretrained', 'dpn92', pretrained=True)
self.head = DetectionHead(2688, num_classes)
def forward(self, x):
x = self.dpn92.features(x)
x = F.avg_pool2d(x, kernel_size=7, stride=1)
x = x.view(x.size(0), -1)
x = self.head(x)
return x
# Define the class names
class_names = ['class0', 'class1', 'class2', 'class3', 'class4']
# Load the model and the weights
model = DPN92Detection(num_classes=len(class_names))
model.load_state_dict(torch.load('DPN92_extra_5k.pth.tar', map_location='cpu')['state_dict'])
# Set the model to evaluation mode
model.eval()
# Define the image transformer
image_transforms = transforms.Compose([
transforms.ToPILImage(),
transforms.Resize((224, 224)),
transforms.ToTensor(),
transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225]),
])
# Load the image
image = cv2.imread('test.jpg')
# Transform the image
input_image = image_transforms(image)
input_image = input_image.unsqueeze(0)
# Make a prediction
with torch.no_grad():
output = model(input_image)
# Get the class probabilities
class_probs = F.softmax(output[:, :len(class_names)], dim=1)
# Get the bounding box coordinates, sizes and class indices
coords_sizes_classes = output[:, len(class_names):].view(-1, 5)
coords_sizes_classes[:, :2] = torch.sigmoid(coords_sizes_classes[:, :2])
coords_sizes_classes[:, 2] = torch.exp(coords_sizes_classes[:, 2])
coords_sizes_classes[:, 3:5] = torch.argmax(coords_sizes_classes[:, 3:], dim=1).unsqueeze(1)
coords_sizes_classes = coords_sizes_classes.cpu().numpy()
# Filter out the boxes with low confidence
conf_threshold = 0.5
filtered_boxes = coords_sizes_classes[class_probs[0] > conf_threshold]
# Draw the boxes on the image
for box in filtered_boxes:
x, y, w, h, c = box
x *= image.shape[1]
y *= image.shape[0]
w *= image.shape[1]
h *= image.shape[0]
x1 = int(x - w / 2)
y1 = int(y - h / 2)
x2 = int(x + w / 2)
y2 = int(y + h / 2)
cv2.rectangle(image, (x1, y1), (x2, y2), (0, 255, 0), 2)
cv2.putText(image, class_names[int(c)], (x1, y1 - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
# Show the image
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的代码中,我们定义了一个名为`DetectionHead`的自定义头,用于检测图像中的对象,并输出它们的坐标、大小和类别。然后,我们定义了一个名为`DPN92Detection`的模型,该模型使用DPN92预训练模型和自定义头进行图像检测和分类。我们还定义了一些变量,如类名、图像变换器、置信度阈值等。最后,我们将模型和权重加载到内存中,并使用`cv2`库加载图像。我们将图像传递给模型,然后使用`softmax`函数获取类别概率,使用`sigmoid`和`exp`函数获取边界框的坐标和大小,并使用`argmax`函数获取类别索引。最后,我们过滤掉低置信度的边界框,并将它们绘制在原始图像上。
基于dpn模型实现目标检测
DPN (Dual Path Network) 是一种深度卷积神经网络,可以用于图像分类和目标检测任务。下面是基于 DPN 模型实现目标检测的步骤:
1. 数据准备:收集并标注训练数据,包括目标类别和位置信息。同时也要准备测试数据。
2. 模型选择:选择适合目标检测任务的 DPN 模型,可以选择已有的预训练模型或者自己训练模型。
3. 数据预处理:对训练和测试数据进行预处理,包括缩放、裁剪和数据增强等操作,以提高模型的鲁棒性和泛化能力。
4. 模型训练:使用标注好的训练数据对模型进行训练,并不断调整模型的参数以提高性能。
5. 模型测试:使用测试数据对训练好的模型进行测试,并评估模型的性能指标,如精度、召回率、F1 值等。
6. 模型优化:根据测试结果对模型进行优化,包括调整模型结构、训练参数和数据预处理等。
7. 部署和应用:将训练好的模型部署到实际应用中,例如通过 API 接口提供目标检测服务,或集成到其他系统中。
需要注意的是,在实际应用中,还需要考虑模型的速度和内存占用等因素,以提高系统的响应速度和稳定性。
阅读全文
相关推荐
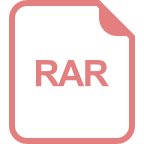
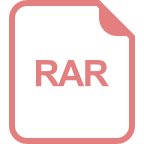
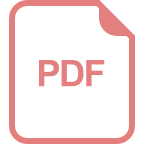
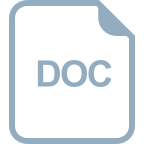
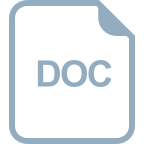
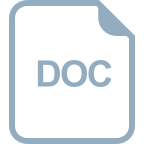





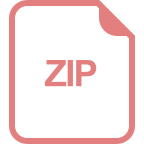
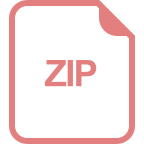
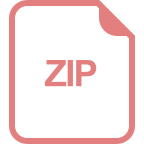