keras实现resnet18对四种天气分类,并绘制图像
时间: 2023-07-11 11:07:12 浏览: 55
首先,我们需要准备数据集。这里我们可以使用Kaggle上的一个天气分类数据集,包含四种天气:晴天、多云、雨天和雪天。可以在此链接中找到数据集:https://www.kaggle.com/c/6826/download-all。
接下来,我们需要安装Keras库。可以在终端中使用以下命令进行安装:
```
pip install keras
```
然后,我们可以开始编写代码。以下是一个可以实现resnet18对四种天气分类的Keras代码,并且绘制出了模型的训练和验证曲线图像:
```
import tensorflow as tf
from tensorflow.keras.layers import Input, Conv2D, MaxPooling2D, Dense, Flatten, Dropout
from tensorflow.keras.models import Model
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.preprocessing.image import ImageDataGenerator
import matplotlib.pyplot as plt
# 设置输入图像的大小和通道数
input_shape = (224, 224, 3)
# 构建ResNet18模型
def resnet_block(inputs, num_filters, kernel_size, strides, activation='relu'):
x = Conv2D(num_filters, kernel_size=kernel_size, strides=strides, padding='same')(inputs)
x = BatchNormalization()(x)
x = Activation(activation)(x)
x = Conv2D(num_filters, kernel_size=kernel_size, padding='same')(x)
x = BatchNormalization()(x)
# shortcut
shortcut = Conv2D(num_filters, kernel_size=1, strides=strides)(inputs)
shortcut = BatchNormalization()(shortcut)
x = Add()([x, shortcut])
x = Activation(activation)(x)
return x
def resnet18(input_shape, num_classes):
inputs = Input(shape=input_shape)
x = Conv2D(64, kernel_size=7, strides=2, padding='same')(inputs)
x = BatchNormalization()(x)
x = Activation('relu')(x)
x = MaxPooling2D(pool_size=3, strides=2, padding='same')(x)
# resnet blocks
x = resnet_block(x, 64, 3, 1)
x = resnet_block(x, 64, 3, 1)
x = resnet_block(x, 128, 3, 2)
x = resnet_block(x, 128, 3, 1)
x = resnet_block(x, 256, 3, 2)
x = resnet_block(x, 256, 3, 1)
x = resnet_block(x, 512, 3, 2)
x = resnet_block(x, 512, 3, 1)
x = AveragePooling2D(pool_size=7)(x)
x = Flatten()(x)
x = Dense(num_classes, activation='softmax')(x)
model = Model(inputs=inputs, outputs=x)
return model
# 初始化模型和超参数
model = resnet18(input_shape=input_shape, num_classes=4)
optimizer = Adam(lr=0.001)
model.compile(loss='categorical_crossentropy', optimizer=optimizer, metrics=['accuracy'])
# 加载数据集
train_datagen = ImageDataGenerator(rescale=1./255, shear_range=0.2, zoom_range=0.2, horizontal_flip=True)
test_datagen = ImageDataGenerator(rescale=1./255)
train_generator = train_datagen.flow_from_directory('weather/train', target_size=(224, 224), batch_size=32, class_mode='categorical')
validation_generator = test_datagen.flow_from_directory('weather/validation', target_size=(224, 224), batch_size=32, class_mode='categorical')
# 训练模型
history = model.fit(train_generator, steps_per_epoch=len(train_generator), epochs=10, validation_data=validation_generator, validation_steps=len(validation_generator))
# 绘制训练和验证曲线
plt.plot(history.history['accuracy'], label='training accuracy')
plt.plot(history.history['val_accuracy'], label='validation accuracy')
plt.title('Training and Validation Accuracy')
plt.xlabel('Epoch')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
plt.plot(history.history['loss'], label='training loss')
plt.plot(history.history['val_loss'], label='validation loss')
plt.title('Training and Validation Loss')
plt.xlabel('Epoch')
plt.ylabel('Loss')
plt.legend()
plt.show()
```
解释一下以上代码的主要部分:
- `resnet_block()` 函数:这个函数实现了ResNet18的基本块。它接受输入张量,然后通过两个卷积层实现一个残差块,并在最后使用了一个shortcut连接。
- `resnet18()` 函数:这个函数使用了上述的基本块来构建整个ResNet18模型。它接受输入的形状和分类数目,并返回构建好的模型。
- `model.compile()` 函数:这个函数设置了模型的损失函数、优化器和评价指标。
- `ImageDataGenerator` 类:这个类实现了对数据集进行增强的功能,在训练模型时可以使用它来增加数据量、减少过拟合等。
- `model.fit()` 函数:这个函数开始训练模型,并返回一个 `history` 对象,其中包含了训练过程中的各种指标和损失值。
- `plt.plot()` 函数:这个函数用于绘制训练和验证曲线。
最后,我们可以运行以上代码来训练模型并绘制曲线。
相关推荐
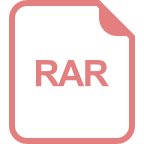
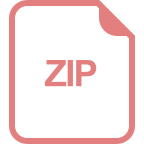











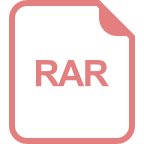
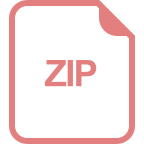
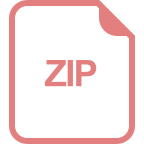