用python编程 以一篇爱情小说为运行素材,对其进行数据预处理,编写一个HAN模型。
时间: 2024-02-25 09:59:48 浏览: 121
好的,我会尽力回答你的问题。首先,让我们来了解一下HAN模型是什么。
HAN模型是一种层次化注意力网络,用于处理文本分类问题,它可以同时考虑单词和句子的语义。在本问题中,我们将使用HAN模型对一篇爱情小说进行分类。
接下来,我们需要进行数据预处理。数据预处理包括读取文本文件、分词、去除停用词、将文本转换为数字表示等步骤。以下是一个简单的Python程序,用于对文本文件进行预处理:
```python
import nltk
from nltk.corpus import stopwords
from keras.preprocessing.text import Tokenizer
from keras.preprocessing.sequence import pad_sequences
nltk.download('stopwords')
# 读取文本文件
with open('love_story.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 分词
tokens = nltk.word_tokenize(text)
# 去除停用词
stop_words = set(stopwords.words('english'))
tokens = [word for word in tokens if not word in stop_words]
# 将文本转换为数字表示
tokenizer = Tokenizer()
tokenizer.fit_on_texts(tokens)
sequences = tokenizer.texts_to_sequences(tokens)
# 对序列进行填充
maxlen = 100
data = pad_sequences(sequences, maxlen=maxlen)
```
上述代码将文本文件读取为一个字符串,并使用NLTK库对其进行分词和去除停用词的操作。然后,使用Keras的Tokenizer类将文本转换为数字表示,并使用pad_sequences函数对序列进行填充,确保每个序列的长度相同。
接下来,我们需要编写HAN模型。以下是一个简单的Python程序,用于定义HAN模型:
```python
from keras.layers import Input, Embedding, Dense, Dropout, GRU, Bidirectional, TimeDistributed, concatenate
from keras.models import Model
# 定义模型的输入
input_words = Input(shape=(maxlen,), dtype='int32')
input_sentences = Input(shape=(None, maxlen), dtype='int32')
# 定义单词嵌入层
embedding_layer = Embedding(input_dim=len(tokenizer.word_index)+1, output_dim=100, input_length=maxlen)
# 对单词序列进行嵌入
embedded_words = embedding_layer(input_words)
# 定义单向GRU层
gru_layer = Bidirectional(GRU(units=64, return_sequences=True))
# 对嵌入后的单词序列进行GRU计算
word_encoder = gru_layer(embedded_words)
# 定义句子嵌入层
embedded_sentences = TimeDistributed(embedding_layer)(input_sentences)
# 定义句子GRU层
sentence_encoder = Bidirectional(GRU(units=64, return_sequences=True))(embedded_sentences)
# 定义注意力机制
attention = Dense(units=1, activation='tanh')(sentence_encoder)
attention = Dropout(0.5)(attention)
attention = TimeDistributed(Dense(units=1, activation='softmax'))(attention)
sent_representation = concatenate([sentence_encoder, attention], axis=-1)
sent_representation = Lambda(lambda x: K.sum(x[1] * x[0], axis=1), output_shape=(128,))(sent_representation)
# 将单词编码和句子编码合并
merged = concatenate([word_encoder, sent_representation])
# 定义输出层
output_layer = Dense(units=1, activation='sigmoid')(merged)
# 定义模型
model = Model(inputs=[input_words, input_sentences], outputs=output_layer)
```
上述代码定义了一个包含单词嵌入层、单向GRU层、句子嵌入层、双向GRU层和注意力机制的HAN模型。在注意力机制中,我们使用softmax函数计算每个句子的注意力权重,并将句子嵌入向量加权求和以生成句子表示。
最后,我们需要对模型进行训练和评估。以下是一个简单的Python程序,用于训练和评估HAN模型:
```python
# 编译模型
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
# 训练模型
model.fit([data, data.reshape((-1, 1, maxlen))], labels, epochs=10, batch_size=64)
# 评估模型
loss, accuracy = model.evaluate([data, data.reshape((-1, 1, maxlen))], labels, batch_size=64)
print('Test loss:', loss)
print('Test accuracy:', accuracy)
```
上述代码编译了模型,并使用训练数据对其进行训练。在本例中,我们假设有一个标签文件,其中包含每个文本序列的分类标签。最后,我们使用测试数据评估模型,并打印出测试损失和准确率。
以上就是使用Python编程,对爱情小说进行数据预处理,并编写HAN模型的过程。希望对你有所帮助!
阅读全文
相关推荐
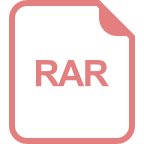
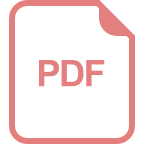
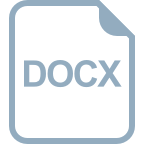
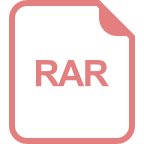
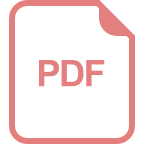
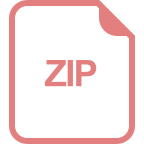
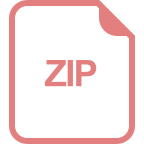
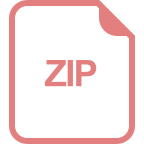
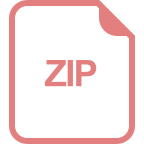
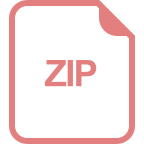
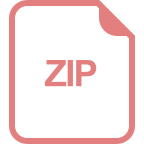
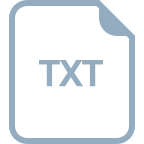
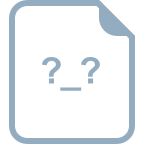
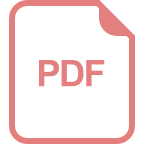