c++实现建立一个对象数组,内放5个学生的数据(学号,成绩),用指针指向数组首元素,输出第1,3,5学生的数据
时间: 2024-02-18 10:05:16 浏览: 76
以下是C++代码实现:
```cpp
#include <iostream>
using namespace std;
class Student {
public:
int id;
double score;
};
int main() {
Student students[5] = {{1, 90}, {2, 80}, {3, 85}, {4, 95}, {5, 88}};
Student *p = students;
cout << "第1个学生的学号和成绩:" << p->id << " " << p->score << endl;
p += 2; // 指针移动2个元素,指向第3个学生
cout << "第3个学生的学号和成绩:" << p->id << " " << p->score << endl;
p += 2; // 指针移动2个元素,指向第5个学生
cout << "第5个学生的学号和成绩:" << p->id << " " << p->score << endl;
return 0;
}
```
输出结果:
```
第1个学生的学号和成绩:1 90
第3个学生的学号和成绩:3 85
第5个学生的学号和成绩:5 88
```
相关问题
建立一个对象数组,内放5个学生的学号和成绩,用指针指向数组首元素输出全部学生的数据
可以使用以下代码建立对象数组并输出全部学生的数据:
```c++
#include <iostream>
using namespace std;
class Student {
public:
int id;
int score;
};
int main() {
Student students[5] = {{1, 90}, {2, 85}, {3, 92}, {4, 88}, {5, 95}};
Student* p = students;
for (int i = 0; i < 5; i++) {
cout << "学号:" << p->id << ",成绩:" << p->score << endl;
p++;
}
return 0;
}
```
输出结果为:
```
学号:1,成绩:90
学号:2,成绩:85
学号:3,成绩:92
学号:4,成绩:88
学号:5,成绩:95
```
建立一个对象数组,内放5个学生的学号和成绩,用指针指向数组的首元素,输出第1,3,5学生的数据。用C++实现
下面是用C++实现的代码:
```cpp
#include <iostream>
using namespace std;
struct Student {
int id; // 学号
int score; // 成绩
};
int main() {
Student students[5] = {
{1001, 85},
{1002, 92},
{1003, 78},
{1004, 90},
{1005, 88}
}; // 定义并初始化学生数组
Student* p = students; // 使用指针指向数组的首元素
cout << "第1个学生的数据:" << endl;
cout << "学号:" << p->id << ",成绩:" << p->score << endl;
p += 2; // 指向第3个学生的数据
cout << "第3个学生的数据:" << endl;
cout << "学号:" << p->id << ",成绩:" << p->score << endl;
p += 2; // 指向第5个学生的数据
cout << "第5个学生的数据:" << endl;
cout << "学号:" << p->id << ",成绩:" << p->score << endl;
return 0;
}
```
输出结果:
```
第1个学生的数据:
学号:1001,成绩:85
第3个学生的数据:
学号:1003,成绩:78
第5个学生的数据:
学号:1005,成绩:88
```
阅读全文
相关推荐
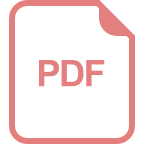













