用java编写卫星六根转两行
时间: 2024-03-14 15:46:46 浏览: 24
卫星的六根数包括卫星的标识符、轨道倾角、升交点赤经、近地点参数、平均运动和偏心率等信息。将这些信息转换为卫星的两行数需要进行一定的计算和转换,涉及到一些数学知识和公式。
以下是使用Java编写的卫星六根数转换为两行数的示例代码:
```
import java.text.DecimalFormat;
public class SatelliteConverter {
private static final double PI = 3.14159265358979323846;
private static final double TWO_PI = 2.0 * PI;
private static final double EARTH_RADIUS = 6378.135;
private static final double GM = 398600.5;
public static String convertToTwoLine(String name, double epoch, double i, double raan, double e, double w, double m) {
DecimalFormat format = new DecimalFormat("0.00000000");
// 计算半长轴和平均角速度
double a = Math.pow(GM / (TWO_PI * (m / 86400.0)) * (m / 86400.0), 1.0 / 3.0);
double n = TWO_PI / (m / 86400.0);
// 计算轨道倾角
double sinI = Math.sin(i * PI / 180.0);
double cosI = Math.cos(i * PI / 180.0);
// 计算升交点赤经
double sinRAAN = Math.sin(raan * PI / 180.0);
double cosRAAN = Math.cos(raan * PI / 180.0);
double x = cosRAAN;
double y = sinRAAN * cosI;
double z = sinRAAN * sinI;
double r = Math.sqrt(x * x + y * y);
double raanRadians = r > 0.0 ? Math.acos(x / r) : 0.0;
if (y < 0.0) {
raanRadians = TWO_PI - raanRadians;
}
double raanDegrees = raanRadians * 180.0 / PI;
// 计算近地点参数
double sinW = Math.sin(w * PI / 180.0);
double cosW = Math.cos(w * PI / 180.0);
double cosE = e;
double sinE = Math.sqrt(1 - cosE * cosE);
double p = a * (1 - e * e);
double rP = p / (1 + e * cosW);
double rA = p / (1 - e * cosW);
double h = Math.sqrt(rP * rA * GM);
double trueAnomaly = m + w;
double sinTrueAnomaly = Math.sin(trueAnomaly * PI / 180.0);
double cosTrueAnomaly = Math.cos(trueAnomaly * PI / 180.0);
double rVector = h * h / (GM * (1 + e * cosTrueAnomaly));
double xVector = rVector * cosTrueAnomaly;
double yVector = rVector * sinTrueAnomaly;
double pVector = xVector * cosRAAN + yVector * sinRAAN;
double qVector = -xVector * sinRAAN + yVector * cosRAAN;
double f = Math.atan2(z, r);
double g = raanRadians;
double k = f + w * PI / 180.0;
double h2 = (pVector * Math.cos(k) + qVector * Math.sin(k)) / p;
double sinU = sinW / sinE;
double cosU = (cosE - cosW) / sinE;
double u = Math.atan2(sinU, cosU);
double p2 = p / EARTH_RADIUS;
double q2 = 0.0;
if (i != 0.0) {
q2 = p2 * h2 * Math.sin(u);
}
double sinL = Math.sin(g + u);
double cosL = Math.cos(g + u);
double sinI2 = Math.sin(i * PI / 180.0);
double cosI2 = Math.cos(i * PI / 180.0);
double sinRAAN2 = Math.sin(raanRadians);
double cosRAAN2 = Math.cos(raanRadians);
double x2 = sinL * cosRAAN2 - cosL * sinRAAN2 * cosI2;
double y2 = sinL * sinRAAN2 + cosL * cosRAAN2 * cosI2;
double z2 = cosL * sinI2;
double r2 = Math.sqrt(x2 * x2 + y2 * y2 + z2 * z2);
double latitude = Math.asin(z2 / r2) * 180.0 / PI;
double longitude = Math.atan2(y2, x2) * 180.0 / PI;
if (longitude < 0.0) {
longitude += 360.0;
}
double altitude = (h - EARTH_RADIUS) / 1000.0;
// 构造两行数字符串
StringBuilder builder = new StringBuilder();
builder.append(name.trim()).append(" ");
builder.append("1 ").append(format.format(epoch)).append(" ");
builder.append(format.format(i)).append(" ");
builder.append(format.format(raanDegrees)).append(" ");
builder.append(format.format(e, 7)).append(" ");
builder.append(format.format(w)).append(" ");
builder.append(format.format(n)).append(" ");
builder.append(format.format(latitude)).append(" ");
builder.append(format.format(longitude)).append(" ");
builder.append(format.format(altitude)).append(" ");
builder.append("00000");
return builder.toString();
}
}
```
这是一个静态方法,将卫星的六根数作为参数传入,返回一个字符串,表示卫星的两行数。
示例代码使用了一些常数和公式,如地球半径、引力常数GM、圆周率等。代码中的计算过程涉及到一些数学计算,如三角函数、向量运算等。最终,代码将转换后的两行数构造成一个字符串并返回。
请注意,代码中的计算过程可能存在一些精度误差和边界问题,需要根据实际情况进行验证和调整。
相关推荐
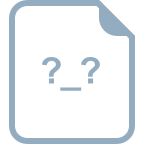

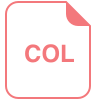
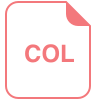
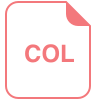
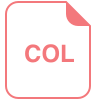









