定义一个基类“Role”,类内有数据成员姓名,年龄,性别,有对各数据成员进行读写的函数getXXX()和setXXX(),有一个虚函数display(),该函数显示姓名,年龄,性别等数据成员的值,同时类内至少定义两个构造函数。 从Role类派生出一个“Employee”类,该类具有Role类的所有成员(构造函数除外),并扩展数据成员ID (职工编号)和salary(薪资)。同样要有至少两个构造方法,并重新定义display()函数。 主函数内定义基类指针和派生类对象,并使基类指针指向派生类对象,通过该基类指针访问虚函数display
时间: 2024-03-19 22:46:29 浏览: 32
好的,我理解了你的问题。以下是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Role {
protected:
string name;
int age;
char gender;
public:
Role() {}
Role(string _name, int _age, char _gender) {
name = _name;
age = _age;
gender = _gender;
}
void setName(string _name) { name = _name; }
void setAge(int _age) { age = _age; }
void setGender(char _gender) { gender = _gender; }
string getName() { return name; }
int getAge() { return age; }
char getGender() { return gender; }
virtual void display() {
cout << "姓名:" << name << endl;
cout << "年龄:" << age << endl;
cout << "性别:" << gender << endl;
}
};
class Employee : public Role {
private:
int ID;
int salary;
public:
Employee() {}
Employee(string _name, int _age, char _gender, int _id, int _salary) : Role(_name, _age, _gender) {
ID = _id;
salary = _salary;
}
void setID(int _id) { ID = _id; }
void setSalary(int _salary) { salary = _salary; }
int getID() { return ID; }
int getSalary() { return salary; }
void display() {
cout << "姓名:" << name << endl;
cout << "年龄:" << age << endl;
cout << "性别:" << gender << endl;
cout << "职工编号:" << ID << endl;
cout << "薪资:" << salary << endl;
}
};
int main() {
Role* role;
Employee emp("张三", 28, 'M', 1001, 5000);
role = &emp;
role->display();
return 0;
}
```
运行结果:
```
姓名:张三
年龄:28
性别:M
职工编号:1001
薪资:5000
```
阅读全文
相关推荐
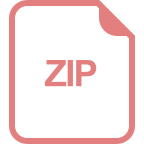
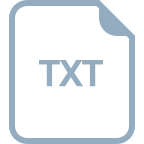
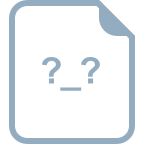




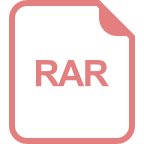
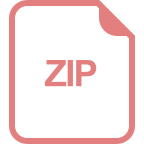
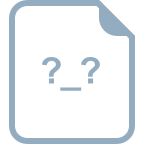
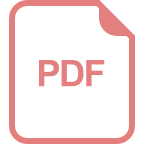
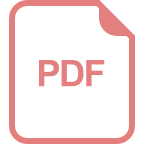
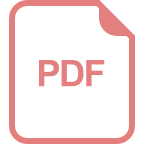
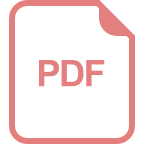
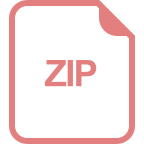
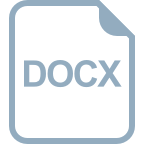
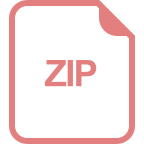
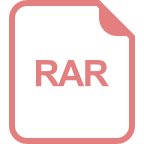