php实现svn代码量统计
时间: 2023-07-11 21:26:13 浏览: 71
您可以使用PHP的SVN库来连接到SVN服务器并获取代码库的统计信息。以下是一个示例代码,可用于获取某个版本号的代码量统计信息:
```php
<?php
// SVN服务器设置
$svn_server = "svn://svn.example.com/myproject";
$svn_username = "username";
$svn_password = "password";
// 获取SVN库
$svn = svn_connect($svn_server);
svn_auth_set_parameter(SVN_AUTH_PARAM_DEFAULT_USERNAME, $svn_username);
svn_auth_set_parameter(SVN_AUTH_PARAM_DEFAULT_PASSWORD, $svn_password);
// 指定版本号
$revision = 1234;
// 统计代码量
$command = "svn diff -r " . ($revision - 1) . ":" . $revision . " " . $svn_server . " --summarize";
$output = shell_exec($command);
// 解析输出结果
$lines_added = 0;
$lines_deleted = 0;
$files_changed = 0;
$lines = explode("\n", $output);
foreach ($lines as $line) {
if (preg_match('/^[ADUMR]\s+(.*)$/', $line, $matches)) {
$files_changed++;
$file = $matches[1];
if (preg_match('/\.php$/', $file)) {
$command = "svn diff -x -w -r " . ($revision - 1) . ":" . $revision . " " . $svn_server . "/" . $file . " | grep -E '^[+|\-]' | wc -l";
$output = trim(shell_exec($command));
$lines_added += (int) $output;
$command = "svn diff -x -w -r " . ($revision - 1) . ":" . $revision . " " . $svn_server . "/" . $file . " | grep -E '^\-' | wc -l";
$output = trim(shell_exec($command));
$lines_deleted += (int) $output;
}
}
}
// 输出统计信息
echo "Files changed: " . $files_changed . "\n";
echo "Lines added: " . $lines_added . "\n";
echo "Lines deleted: " . $lines_deleted . "\n";
?>
```
在以上代码中,首先需要设置SVN服务器的地址、用户名和密码。然后,使用`svn_connect()`函数连接到SVN服务器,并使用`svn_auth_set_parameter()`函数设置用户名和密码。接下来,使用`svn diff`命令获取指定版本号的代码库变更信息,并使用`shell_exec()`函数执行该命令。然后,解析输出结果,统计代码量并输出统计信息。
在解析输出结果时,使用了正则表达式来匹配文件路径和变更类型,并使用`svn diff`命令获取指定文件的代码变更信息。然后,使用`grep`命令和正则表达式来匹配添加和删除的行,并使用`wc -l`命令统计行数。最后,累加添加和删除的行数,并输出统计信息。
相关推荐
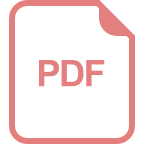
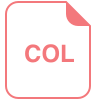













