hanlp重复二分文本聚类代码
时间: 2023-07-09 11:45:28 浏览: 220
以下是使用 HanLP 和 scikit-learn 实现的重复二分文本聚类代码,具体实现思路为:
1. 使用 HanLP 进行中文分词,得到文本的词语列表。
2. 使用 TF-IDF 对文本进行特征提取。
3. 使用重复二分 K-Means 算法进行文本聚类。
```python
from pyhanlp import *
import numpy as np
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.cluster import KMeans
from sklearn.metrics.pairwise import cosine_similarity
# 设置分词器
tokenizer = JClass("com.hankcs.hanlp.tokenizer.StandardTokenizer")
# 加载停用词
with open('stopwords.txt', 'r', encoding='utf-8') as f:
stopwords = set([line.strip() for line in f])
# 对文本进行分词
def segment(text):
words = [word.word for word in tokenizer.segment(text)]
words = [word for word in words if word not in stopwords]
return words
# 重复二分 K-Means 算法
def repeated_bisection_kmeans(X, k, n_repeats=10):
best_labels = None
best_inertia = np.inf
for i in range(n_repeats):
labels = KMeans(n_clusters=k).fit_predict(X)
centroids = np.zeros((k, X.shape[1]))
for j in range(k):
centroids[j] = np.mean(X[labels == j], axis=0)
sim = cosine_similarity(X, centroids)
inertia = sum([max(sim[i]) for i in range(X.shape[0])])
if inertia < best_inertia:
best_labels = labels
best_inertia = inertia
return best_labels
# 加载文本数据
with open('data.txt', 'r', encoding='utf-8') as f:
texts = [line.strip() for line in f]
# 对文本进行分词
segments = [segment(text) for text in texts]
# 计算 TF-IDF 特征
vectorizer = TfidfVectorizer(tokenizer=lambda x: x, lowercase=False)
X = vectorizer.fit_transform(segments).toarray()
# 进行文本聚类
k = 3 # 聚成3类
labels = repeated_bisection_kmeans(X, k)
# 输出聚类结果
for i in range(k):
print('Cluster %d:' % i)
for j in range(len(texts)):
if labels[j] == i:
print(texts[j])
print('==================================')
```
需要注意的是,代码中使用了一个停用词列表,可以根据实际情况调整停用词列表。此外,代码中的文本数据需要以一行一条的形式保存在 data.txt 文件中。
阅读全文
相关推荐
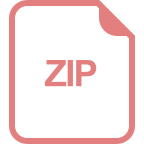
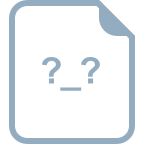
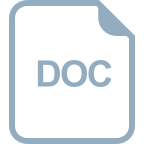
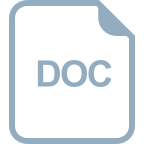
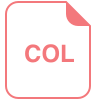
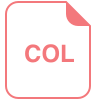
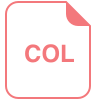
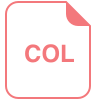
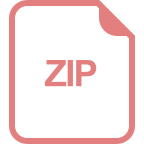
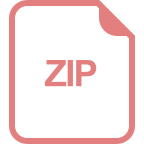
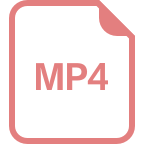
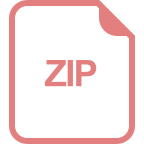
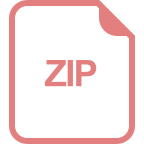
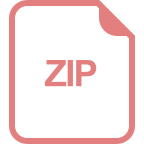
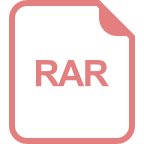
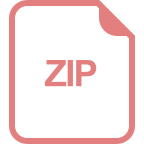