eclipse搭建Spring Boot+Spring Cloud微服务
时间: 2024-01-23 20:03:26 浏览: 230
好的,下面是搭建Spring Boot+Spring Cloud微服务的步骤:
1. 安装Java开发环境和Maven构建工具;
2. 在eclipse中安装Spring Boot和Spring Cloud插件;
3. 创建一个Spring Boot工程作为微服务的注册中心,使用Eureka或Consul等服务注册中心;
4. 创建其他的Spring Boot工程作为微服务提供者或消费者,使用Feign或Ribbon等负载均衡的方式进行服务调用;
5. 在每个微服务工程中配置Spring Cloud的相关组件,如Config、Zuul等;
6. 部署微服务到云平台,如Docker、Kubernetes等。
以上就是基本的搭建步骤,当然具体的实现过程可能会有些细节上的差异。希望这些信息能够对您有所帮助,如果您还有其他问题,可以随时问我。
相关问题
eclipse搭建Spring Boot+Spring Cloud微服务代码
好的,下面是一个简单的示例代码,演示如何在eclipse中搭建Spring Boot+Spring Cloud微服务:
1. 注册中心(Eureka Server):
```java
@SpringBootApplication
@EnableEurekaServer
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
```
2. 微服务提供者:
```java
@SpringBootApplication
@EnableDiscoveryClient
public class ProductServiceApplication {
public static void main(String[] args) {
SpringApplication.run(ProductServiceApplication.class, args);
}
@RestController
class ProductController {
@GetMapping("/products/{id}")
public String getProductById(@PathVariable Long id) {
return "Product Id: " + id;
}
}
}
```
3. 微服务消费者:
```java
@SpringBootApplication
@EnableDiscoveryClient
@EnableFeignClients
public class OrderServiceApplication {
public static void main(String[] args) {
SpringApplication.run(OrderServiceApplication.class, args);
}
@Autowired
private ProductServiceClient productServiceClient;
@RestController
class OrderController {
@GetMapping("/orders/{id}")
public String getOrderById(@PathVariable Long id) {
String product = productServiceClient.getProductById(id);
return "Order Id: " + id + ", Product: " + product;
}
}
}
@FeignClient("product-service") // 与提供者应用的spring.application.name相同
interface ProductServiceClient {
@GetMapping("/products/{id}")
String getProductById(@PathVariable("id") Long id);
}
```
以上代码仅供参考,实际开发中可能会更加复杂。希望这些信息能够对您有所帮助,如果您还有其他问题,可以随时问我。
eclipse 使用 spring cloud alibaba + spring boot 创建项目
下面是使用 Eclipse 创建 Spring Cloud Alibaba + Spring Boot 项目的步骤:
1. 打开 Eclipse,选择 File -> New -> Spring Starter Project。
2. 在 New Spring Starter Project 对话框中,填写以下项目信息:
- Name:项目名称。
- Type:选择 Maven 项目。
- Packaging:选择 Jar。
- Java Version:选择 Java 8 或更高版本。
- Spring Boot Version:选择最新版本。
- Group:项目的 Group ID。
- Artifact:项目的 Artifact ID。
- Dependencies:选择 Spring Web、Spring Cloud Alibaba Nacos Config、Spring Cloud Alibaba Sentinel 和 Spring Cloud Alibaba Nacos Discovery。
- Description:项目描述。
3. 点击 Next,选择项目的依赖关系和版本,然后点击 Finish。
4. 在项目中添加一个配置文件 application.yml,配置 Nacos 注册中心和 Sentinel 控制台的地址和端口号。
5. 编写代码实现业务逻辑,使用 Spring Cloud Alibaba 提供的组件实现服务注册、配置管理和流量控制等功能。
6. 运行项目,测试业务逻辑是否正常。
以上是使用 Eclipse 创建 Spring Cloud Alibaba + Spring Boot 项目的基本步骤,希望对你有所帮助。
阅读全文
相关推荐
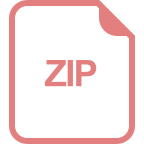
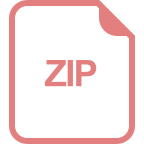
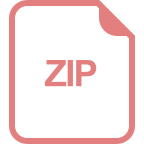
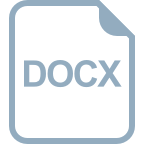
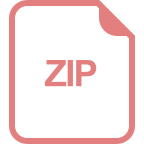
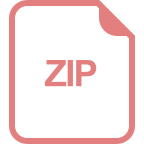
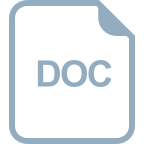
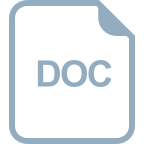
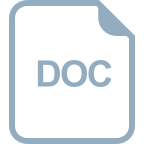
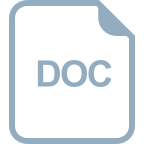
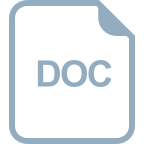
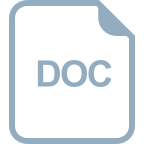
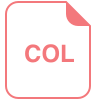
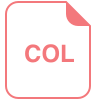
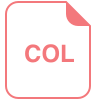