用java 写一个ByteArrayInputStream 转为blob的 TypeHandler
时间: 2023-11-19 15:01:22 浏览: 44
下面是一个示例代码,用于将 Java 的 ByteArrayInputStream 类型转换为 SQL 的 BLOB 类型,可以作为 MyBatis 中的 TypeHandler 使用:
```java
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.sql.Blob;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import org.apache.ibatis.type.BaseTypeHandler;
import org.apache.ibatis.type.JdbcType;
public class ByteArrayInputStreamTypeHandler extends BaseTypeHandler<ByteArrayInputStream> {
@Override
public void setNonNullParameter(PreparedStatement ps, int i, ByteArrayInputStream parameter, JdbcType jdbcType)
throws SQLException {
InputStream inputStream = parameter;
ps.setBlob(i, inputStream);
}
@Override
public ByteArrayInputStream getNullableResult(ResultSet rs, String columnName) throws SQLException {
Blob blob = rs.getBlob(columnName);
InputStream inputStream = null;
if (blob != null) {
try {
inputStream = blob.getBinaryStream();
byte[] bytes = inputStream.readAllBytes();
return new ByteArrayInputStream(bytes);
} catch (IOException e) {
e.printStackTrace();
}
}
return null;
}
@Override
public ByteArrayInputStream getNullableResult(ResultSet rs, int columnIndex) throws SQLException {
Blob blob = rs.getBlob(columnIndex);
InputStream inputStream = null;
if (blob != null) {
try {
inputStream = blob.getBinaryStream();
byte[] bytes = inputStream.readAllBytes();
return new ByteArrayInputStream(bytes);
} catch (IOException e) {
e.printStackTrace();
}
}
return null;
}
@Override
public ByteArrayInputStream getNullableResult(CallableStatement cs, int columnIndex) throws SQLException {
Blob blob = cs.getBlob(columnIndex);
InputStream inputStream = null;
if (blob != null) {
try {
inputStream = blob.getBinaryStream();
byte[] bytes = inputStream.readAllBytes();
return new ByteArrayInputStream(bytes);
} catch (IOException e) {
e.printStackTrace();
}
}
return null;
}
}
```
使用时可以在 MyBatis 的配置文件中添加以下内容:
```xml
<typeHandlers>
<typeHandler javaType="java.io.ByteArrayInputStream" jdbcType="BLOB" handler="com.example.ByteArrayInputStreamTypeHandler" />
</typeHandlers>
```
其中 `javaType` 指定 Java 类型,`jdbcType` 指定 SQL 类型,`handler` 指定 TypeHandler 类的全限定名。
相关推荐
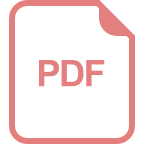
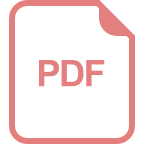
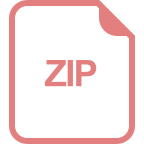














