基于stm32f103超声波模块获取数据程序
时间: 2023-09-26 16:09:07 浏览: 63
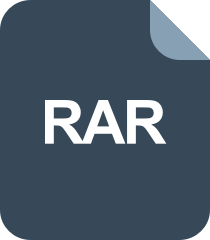
使用stm32f103进行采集
以下是基于STM32F103的超声波模块获取数据的示例程序:
```c
#include "stm32f10x.h"
#define TRIG_PIN GPIO_Pin_12 // 超声波模块的Trig引脚连接到PA12
#define ECHO_PIN GPIO_Pin_11 // 超声波模块的Echo引脚连接到PA11
void delay_us(uint32_t us) // 延时函数,延时us微秒
{
uint32_t i;
for(i=0; i<us*8; i++);
}
int main(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE); // 使能GPIOA时钟
// 配置PA12为输出模式,用于控制超声波模块的Trig引脚
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = TRIG_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置PA11为输入模式,用于接收超声波模块的Echo引脚输出的电平信号
GPIO_InitStructure.GPIO_Pin = ECHO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
while(1)
{
GPIO_ResetBits(GPIOA, TRIG_PIN); // 将Trig引脚拉低
delay_us(2); // 延时2us
GPIO_SetBits(GPIOA, TRIG_PIN); // 将Trig引脚拉高
delay_us(10); // 延时10us
GPIO_ResetBits(GPIOA, TRIG_PIN); // 将Trig引脚拉低
uint32_t pulse_start = 0, pulse_end = 0;
while(GPIO_ReadInputDataBit(GPIOA, ECHO_PIN) == 0); // 等待Echo引脚的电平从低变高
pulse_start = TIM2->CNT; // 记录计数器的值
while(GPIO_ReadInputDataBit(GPIOA, ECHO_PIN) == 1); // 等待Echo引脚的电平从高变低
pulse_end = TIM2->CNT; // 记录计数器的值
uint32_t pulse_duration = pulse_end - pulse_start; // 计算超声波往返时间
float distance = pulse_duration * 0.017; // 计算距离(声速在空气中约为340m/s,每秒传播约17m)
}
}
```
需要注意的是,上述程序中使用了定时器TIM2的计数器来记录Echo引脚电平变化的时间,因此需要在程序中初始化并使能TIM2定时器。此外,还需要根据实际情况调整延时函数delay_us()中的参数,以保证超声波模块的工作正常。
阅读全文
相关推荐
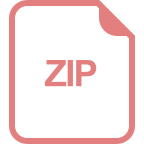
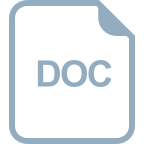
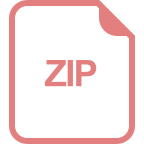
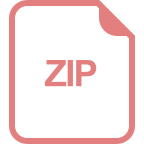
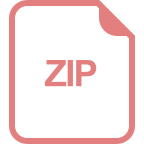
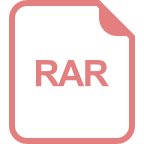
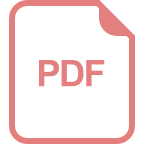
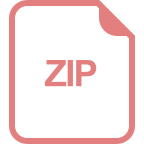
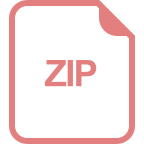
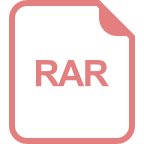
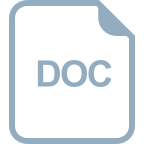
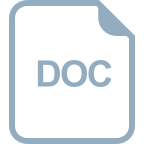

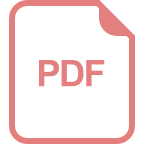
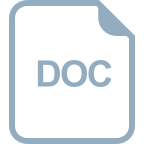
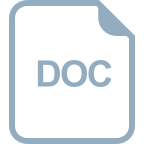
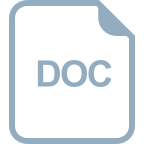