matlab使用pitchshift()函数对语音信号进行变调处理
时间: 2024-03-06 09:51:37 浏览: 467
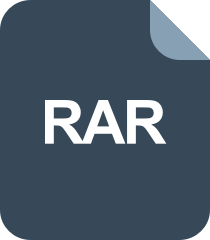
matlab实现语音信号的变调与变速实验
在Matlab中,可以使用pitchshift()函数对语音信号进行变调处理。该函数在Signal Processing Toolbox中提供。要使用pitchshift()函数,可以按照以下步骤进行:
1. 读取原始语音信号文件,并将其转换为数字信号数据。
2. 将数字信号数据传递给pitchshift()函数,并指定要变化的音高或音调的值。
3. 将pitchshift()函数返回的变化后的数字信号数据保存到新的文件中。
下面是一个Matlab示例代码,演示如何使用pitchshift()函数对语音信号进行变调处理:
```matlab
% 读取原始语音信号文件
[audio, Fs] = audioread('original_voice.wav');
% 将数字信号数据传递给pitchshift()函数,并指定要变化的音高或音调的值
pitch_shift = 3; % 将音高升高3个半音
audio_shifted = pitchshift(audio, Fs, pitch_shift);
% 将pitchshift()函数返回的变化后的数字信号数据保存到新的文件中
audiowrite('shifted_voice.wav', audio_shifted, Fs);
```
在上面的代码示例中,我们使用了Matlab中的audioread()函数读取原始语音信号文件,使用pitchshift()函数对信号进行音高变化,然后使用audiowrite()函数将变化后的数字信号数据保存到新的文件中。
阅读全文
相关推荐
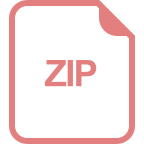
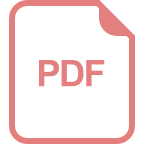
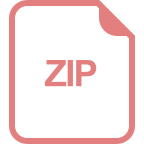
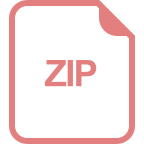
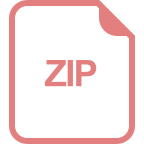
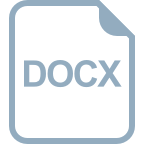









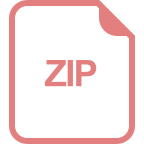
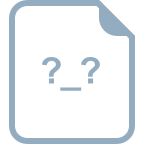