Matlab的代码分别对图像以这四种方法进行提取
时间: 2024-02-17 10:03:34 浏览: 8
好的,下面是Matlab代码实现。假设待处理的图像为img:
1. 原始LBP:
```matlab
function lbp_img = lbp_original(img)
[h, w] = size(img);
lbp_img = zeros(h, w);
for i = 2 : h - 1
for j = 2 : w - 1
center = img(i, j);
code = 0;
code = code + (img(i-1, j-1) >= center) * 2^0;
code = code + (img(i-1, j) >= center) * 2^1;
code = code + (img(i-1, j+1) >= center) * 2^2;
code = code + (img(i, j+1) >= center) * 2^3;
code = code + (img(i+1, j+1) >= center) * 2^4;
code = code + (img(i+1, j) >= center) * 2^5;
code = code + (img(i+1, j-1) >= center) * 2^6;
code = code + (img(i, j-1) >= center) * 2^7;
lbp_img(i, j) = code;
end
end
end
```
2. 圆形LBP:
```matlab
function lbp_img = lbp_circular(img, radius, neighbors)
[h, w] = size(img);
lbp_img = zeros(h, w);
for i = radius + 1 : h - radius
for j = radius + 1 : w - radius
center = img(i, j);
code = 0;
for k = 1 : neighbors
x = i + radius * cos(2 * pi * (k-1) / neighbors);
y = j - radius * sin(2 * pi * (k-1) / neighbors);
if img(round(x), round(y)) >= center
code = code + 2^(k-1);
end
end
lbp_img(i, j) = code;
end
end
end
```
3. 旋转不变LBP:
```matlab
function lbp_img = lbp_rotation_invariant(img, radius, neighbors)
[h, w] = size(img);
lbp_img = zeros(h, w);
for i = radius + 1 : h - radius
for j = radius + 1 : w - radius
center = img(i, j);
code = 0;
for k = 1 : neighbors
x = i + radius * cos(2 * pi * (k-1) / neighbors);
y = j - radius * sin(2 * pi * (k-1) / neighbors);
weight = abs(k - (neighbors+1) / 2);
if img(round(x), round(y)) >= center
code = code + weight * 2^(k-1);
end
end
lbp_img(i, j) = code;
end
end
end
```
4. 旋转不变均匀LBP:
```matlab
function lbp_img = lbp_rotation_invariant_uniform(img, radius, neighbors)
[h, w] = size(img);
lbp_img = zeros(h, w);
for i = radius + 1 : h - radius
for j = radius + 1 : w - radius
center = img(i, j);
code = 0;
for k = 1 : neighbors
x = i + radius * cos(2 * pi * (k-1) / neighbors);
y = j - radius * sin(2 * pi * (k-1) / neighbors);
weight = abs(k - (neighbors+1) / 2);
if img(round(x), round(y)) >= center
code = code + weight * 2^(k-1);
end
end
if is_uniform(code)
lbp_img(i, j) = code;
else
lbp_img(i, j) = 0;
end
end
end
end
function flag = is_uniform(code)
code_bin = dec2bin(code);
code_bin = ['0', repmat('0', 1, 8-length(code_bin)), code_bin];
flag = sum(abs(diff(code_bin))) <= 2;
end
```
其中,is_uniform函数用于判断一个LBP值是否为均匀模式。
相关推荐
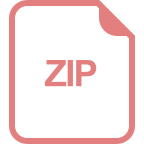
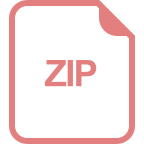
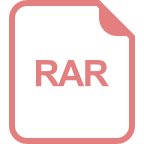
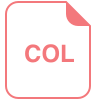
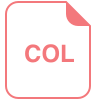
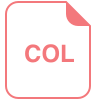
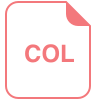
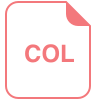









