c语言建立一个链表,每个结点包括姓名和成绩,c语言编程题,程序功能: 建立一个链表,每个结点包括:学号、姓名、性别、年龄,输入一个学号,如果链表中...
时间: 2024-02-13 12:04:38 浏览: 26
好的,这是一个比较复杂的问题,需要较长的代码实现。以下是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义链表节点结构体
typedef struct student {
int id;
char name[20];
int score;
struct student* next;
} Student;
// 定义链表头指针
Student* head = NULL;
// 插入节点函数
void insertNode(int id, char name[], int score) {
Student* newNode = (Student*)malloc(sizeof(Student));
newNode->id = id;
strcpy(newNode->name, name);
newNode->score = score;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
} else {
Student* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 查找节点函数
Student* findNode(int id) {
Student* current = head;
while (current != NULL) {
if (current->id == id) {
return current;
}
current = current->next;
}
return NULL;
}
// 输出链表函数
void printList() {
Student* current = head;
while (current != NULL) {
printf("id: %d, name: %s, score: %d\n", current->id, current->name, current->score);
current = current->next;
}
}
int main() {
// 插入节点
insertNode(1, "Tom", 90);
insertNode(2, "Jerry", 80);
insertNode(3, "Lucy", 70);
// 查找节点并输出结果
int id;
printf("Enter student id to search: ");
scanf("%d", &id);
Student* node = findNode(id);
if (node != NULL) {
printf("id: %d, name: %s, score: %d\n", node->id, node->name, node->score);
} else {
printf("Student not found.\n");
}
// 输出链表
printList();
return 0;
}
```
这个程序会创建一个链表,每个节点包括学号、姓名和成绩。它还会提示用户输入一个学号,然后查找链表中是否有对应的节点,并输出其姓名和成绩。最后,它会输出整个链表的内容。
相关推荐
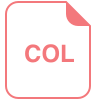
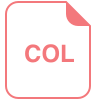
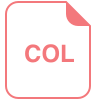
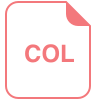
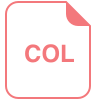









