vc++6.0 win32 五子棋代码
时间: 2023-08-19 16:03:52 浏览: 74
以下是一个简单的VC++ 6.0 Win32五子棋代码示例:
```c++
#include <windows.h>
#define CELL_SIZE 30 // 棋盘格子大小
#define BOARD_SIZE 15 // 棋盘大小
#define WINDOW_WIDTH (CELL_SIZE * BOARD_SIZE + 20)
#define WINDOW_HEIGHT (CELL_SIZE * BOARD_SIZE + 20)
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
WNDCLASS wc = {0};
wc.style = CS_HREDRAW | CS_VREDRAW;
wc.lpfnWndProc = WndProc;
wc.hInstance = hInstance;
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.lpszClassName = TEXT("FiveChessClass");
RegisterClass(&wc);
HWND hwnd = CreateWindow(TEXT("FiveChessClass"), TEXT("Five Chess"), WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, WINDOW_WIDTH, WINDOW_HEIGHT, NULL, NULL, hInstance, NULL);
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
MSG msg = {0};
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
static HBRUSH hWhiteBrush = CreateSolidBrush(RGB(255, 255, 255)); // 白色画刷
static HBRUSH hBlackBrush = CreateSolidBrush(RGB(0, 0, 0)); // 黑色画刷
static int board[BOARD_SIZE][BOARD_SIZE] = {0}; // 棋盘
static int curPlayer = 1; // 当前玩家,1表示黑方,2表示白方
static int winner = 0; // 获胜者,0表示无人获胜,1表示黑方获胜,2表示白方获胜
static BOOL gameOver = FALSE; // 游戏是否结束
switch (msg)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
// 绘制棋盘
for (int i = 0; i < BOARD_SIZE; ++i)
{
for (int j = 0; j < BOARD_SIZE; ++j)
{
RECT rect = {i * CELL_SIZE + 10, j * CELL_SIZE + 10, (i + 1) * CELL_SIZE + 10, (j + 1) * CELL_SIZE + 10};
if (board[i][j] == 1)
{
FillRect(hdc, &rect, hBlackBrush);
}
else if (board[i][j] == 2)
{
FillRect(hdc, &rect, hWhiteBrush);
}
else
{
Rectangle(hdc, rect.left, rect.top, rect.right, rect.bottom);
}
}
}
// 绘制获胜者
if (gameOver)
{
TCHAR szWinner[32] = {0};
if (winner == 1)
{
wsprintf(szWinner, TEXT("Black player wins!"));
}
else if (winner == 2)
{
wsprintf(szWinner, TEXT("White player wins!"));
}
else
{
wsprintf(szWinner, TEXT("Game over!"));
}
TextOut(hdc, 10, CELL_SIZE * BOARD_SIZE + 20, szWinner, lstrlen(szWinner));
}
EndPaint(hwnd, &ps);
break;
}
case WM_LBUTTONDOWN:
{
if (gameOver)
{
break;
}
int x = LOWORD(lParam);
int y = HIWORD(lParam);
if (x < 10 || x > WINDOW_WIDTH - 10 || y < 10 || y > WINDOW_HEIGHT - 10)
{
break;
}
int i = (x - 10) / CELL_SIZE;
int j = (y - 10) / CELL_SIZE;
if (board[i][j] != 0)
{
break;
}
board[i][j] = curPlayer;
// 判断获胜者
int dx[4] = {1, 0, 1, -1};
int dy[4] = {0, 1, 1, 1};
for (int k = 0; k < 4; ++k)
{
int count = 1;
for (int p = 1; p <= 4; ++p)
{
int newi = i + p * dx[k];
int newj = j + p * dy[k];
if (newi < 0 || newi >= BOARD_SIZE || newj < 0 || newj >= BOARD_SIZE || board[newi][newj] != curPlayer)
{
break;
}
++count;
}
for (int p = 1; p <= 4; ++p)
{
int newi = i - p * dx[k];
int newj = j - p * dy[k];
if (newi < 0 || newi >= BOARD_SIZE || newj < 0 || newj >= BOARD_SIZE || board[newi][newj] != curPlayer)
{
break;
}
++count;
}
if (count >= 5)
{
winner = curPlayer;
gameOver = TRUE;
break;
}
}
// 切换玩家
curPlayer = 3 - curPlayer;
// 重新绘制窗口
InvalidateRect(hwnd, NULL, TRUE);
break;
}
default:
return DefWindowProc(hwnd, msg, wParam, lParam);
}
return 0;
}
```
该示例代码使用了Win32 API和GDI进行图形界面的绘制和处理,实现了一个能够双人对战的五子棋游戏。在游戏中会根据玩家的落子情况判断是否获胜,并在游戏结束后显示获胜者。
相关推荐
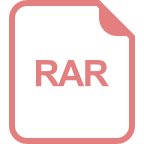
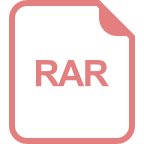














