请用Java的知识,按照以下步骤完成该题:(1)编写LoginFrame类,该类继承Frame实现ActionListener接口。并生成main方法。 (2)编写用户登录界面。 (3)为用户名、密码、提交按钮和重置按钮添加动作事件监听器。 (4)为密码输入框添加键盘监听器,要求只能输入数字和退格键。(调用事件对象的consume()禁止输入字符) (5)为窗口添加监听器,使关闭按钮功能可用。 (6)重写actionPerformed方法,获得事件源对象,并分别处理以下场景事件: 1)判断事件源对象是否为提交或者密码;2)判断事件源对象是否为重置;3)判断事件源对象是否为用户名。 (7)如果事件源对象为提交或者密码,要进行正确性验证和错误事件处理。如果正确,弹出新窗口提示登录成功,如果错误弹出消息框进行提示。 (8)如果事件源对象为重置按钮,要把所有输入框置空。 (9)如果事件源对象为用户名,表明只输入了用户名,未输入密码就回车确认。要把光标自动定位到密码输入框。(密码调用requestFocusInWindow()定位焦点) (10)在main方法中创建LoginFrame对象,运行测试各种事件处理结果是否正确。
时间: 2024-02-19 12:59:01 浏览: 20
很高兴回答您的问题。以下是Java代码实现步骤:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class LoginFrame extends Frame implements ActionListener, KeyListener {
private JLabel userNameLabel, passwordLabel;
private JTextField userNameInput;
private JPasswordField passwordInput;
private JButton submitButton, resetButton;
public LoginFrame() {
initUI();
}
private void initUI() {
this.setSize(400, 200);
this.setLayout(new GridLayout(3, 2));
this.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent we) {
System.exit(0);
}
});
userNameLabel = new JLabel("用户名:");
passwordLabel = new JLabel("密 码:");
userNameInput = new JTextField();
passwordInput = new JPasswordField();
submitButton = new JButton("提交");
resetButton = new JButton("重置");
userNameInput.addKeyListener(this);
submitButton.addActionListener(this);
resetButton.addActionListener(this);
this.add(userNameLabel);
this.add(userNameInput);
this.add(passwordLabel);
this.add(passwordInput);
this.add(submitButton);
this.add(resetButton);
this.setVisible(true);
}
public void actionPerformed(ActionEvent ae) {
Object source = ae.getSource();
if (source == submitButton || source == passwordInput) {
String userName = userNameInput.getText();
String password = new String(passwordInput.getPassword());
if (isValid(userName, password)) {
JOptionPane.showMessageDialog(this, "登录成功!");
this.dispose();
new WelcomeFrame(userName);
} else {
JOptionPane.showMessageDialog(this, "用户名或密码错误!", "错误", JOptionPane.ERROR_MESSAGE);
}
} else if (source == resetButton) {
userNameInput.setText("");
passwordInput.setText("");
} else if (source == userNameInput) {
passwordInput.requestFocusInWindow();
}
}
private boolean isValid(String userName, String password) {
if (userName.equals("admin") && password.equals("123456")) {
return true;
} else {
return false;
}
}
public void keyTyped(KeyEvent ke) {
char c = ke.getKeyChar();
if (!Character.isDigit(c) && c != KeyEvent.VK_BACK_SPACE) {
ke.consume();
}
}
public void keyPressed(KeyEvent ke) {}
public void keyReleased(KeyEvent ke) {}
public static void main(String[] args) {
new LoginFrame();
}
}
class WelcomeFrame extends JFrame {
private JLabel welcomeLabel;
public WelcomeFrame(String userName) {
initUI(userName);
}
private void initUI(String userName) {
this.setSize(300, 150);
this.setLocationRelativeTo(null);
this.setLayout(new FlowLayout());
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
welcomeLabel = new JLabel("欢迎您," + userName + "!");
this.add(welcomeLabel);
this.setVisible(true);
}
}
```
这段代码实现了一个用户登录界面,包含了用户名、密码、提交按钮和重置按钮。在登录时,会进行用户名和密码的正确性验证,如果正确则弹出欢迎窗口,否则弹出错误提示框。在密码输入框中,只能输入数字和退格键,其他字符会被禁止输入。如果只输入了用户名而未输入密码,按回车键将自动定位光标到密码输入框中。
相关推荐
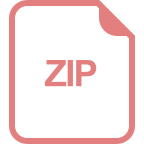
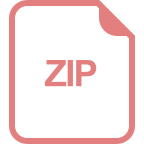














