读取'C:\Users\Qin\Desktop\PYTHON 文件\软工三四班_第一次.txt', 'C:\Users\Qin\Desktop\PYTHON 文件\软工三四班_第二次.txt', 'C:\Users\Qin\Desktop\PYTHON 文件\软工三四班_第三次.txt', 'C:\Users\Qin\Desktop\PYTHON 文件\软工三四班_第四次.txt','C:\Users\Qin\Desktop\PYTHON 文件\软工三四班_第五次.txt'成绩文件,输出每个学生的学号姓名和最高的三次分数
时间: 2023-06-28 09:10:07 浏览: 39
好的,以下是代码实现:
```python
# 定义一个函数用于读取成绩文件
def read_score_file(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
lines = f.readlines()
# 获取每个学生的学号、姓名和成绩
score_dict = {}
for line in lines:
student_info, score_str = line.strip().split(':')
student_id, student_name = student_info.split(',')
scores = list(map(float, score_str.split(',')))
score_dict[student_id] = {'name': student_name, 'scores': scores}
return score_dict
# 定义一个函数用于输出每个学生的学号、姓名和最高的三次分数
def print_top_three_scores(score_dict):
for student_id, info in score_dict.items():
student_name = info['name']
scores = info['scores']
top_three_scores = sorted(scores, reverse=True)[:3]
print(f'{student_id} {student_name}: {top_three_scores}')
# 读取五次成绩文件
score_dict = {}
for i in range(1, 6):
file_path = f'C:\\Users\\Qin\\Desktop\\PYTHON 文件\\软工三四班_第{i}次.txt'
score_dict[i] = read_score_file(file_path)
# 输出每个学生的学号、姓名和最高的三次分数
for student_id in score_dict[1].keys():
student_name = score_dict[1][student_id]['name']
all_scores = []
for i in range(1, 6):
all_scores.extend(score_dict[i][student_id]['scores'])
top_three_scores = sorted(all_scores, reverse=True)[:3]
print(f'{student_id} {student_name}: {top_three_scores}')
```
请注意,这段代码假设每个成绩文件中,每个学生的信息是按照学号、姓名、成绩的顺序排列的,如下所示:
```
180101001,张三:90,80,70,85,92
180101002,李四:85,87,92,76,88
...
```
如果成绩文件的格式不同,需要对代码进行适当修改。
相关推荐
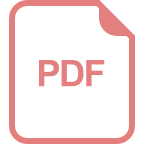
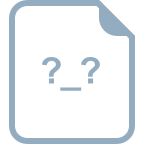
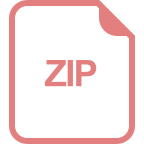
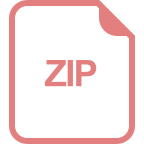
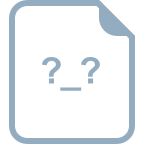
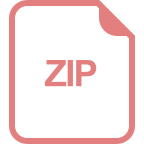
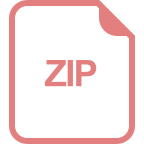
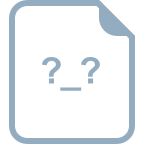
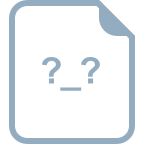
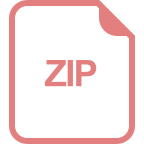
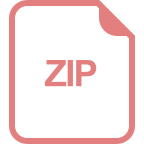
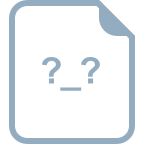
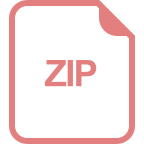
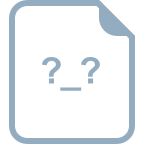