如何在后台线程中更新显示的时间?
时间: 2024-09-13 17:19:35 浏览: 39
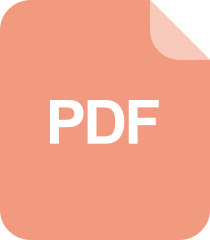
总结Android中多线程更新应用的页面信息的方式
在后台线程中更新显示的时间,通常我们会使用`BackgroundWorker`类或者`Task`和`async`关键字配合。这里以`BackgroundWorker`为例,因为它的设计是为了处理长时间运行的操作而不会阻塞用户界面:
```csharp
using System;
using System.ComponentModel;
using System.Threading.Tasks;
using System.Windows.Forms;
public partial class MainForm : Form
{
private BackgroundWorker backgroundWorker = new BackgroundWorker();
private bool isRunning = false;
private string executionTimeText = "等待中";
public MainForm()
{
InitializeComponent();
backgroundWorker.DoWork += Worker_DoWork;
backgroundWorker.RunWorkerCompleted += Worker_RunWorkerCompleted;
}
private void buttonStart_Click(object sender, EventArgs e)
{
if (!isRunning)
{
isRunning = true;
backgroundWorker.RunWorkerAsync();
buttonStart.Enabled = false;
buttonStop.Enabled = true;
}
}
private void buttonStop_Click(object sender, EventArgs e)
{
isRunning = false;
}
private void Worker_DoWork(object sender, DoWorkEventArgs e)
{
stopwatch.Start();
while (isRunning && stopwatch.IsRunning)
{
// 在这个循环里做你的长时间任务...
Thread.Sleep(100); // 示例:每100毫秒检查一次
// 更新执行时间
if (InvokeRequired)
{
Invoke(new Action(() =>
{
executionTimeText = $"执行时间: {stopwatch.Elapsed.TotalSeconds}秒";
labelExecutionTime.Text = executionTimeText;
}));
}
}
stopwatch.Stop();
}
private void Worker_RunWorkerCompleted(object sender, RunWorkerCompletedEventArgs e)
{
isRunning = false;
buttonStart.Enabled = true;
buttonStop.Enabled = false;
}
}
```
这里,我们在`DoWork`事件中执行长时间任务并在适当的时候调用`Invoke`方法将结果显示到UI线程。当任务完成后,`RunWorkerCompleted`事件会被触发。
阅读全文
相关推荐
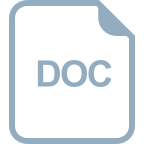
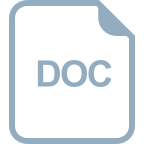
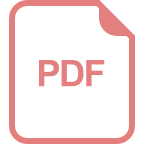
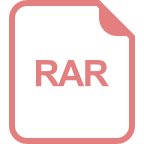
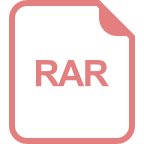
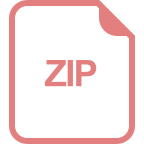
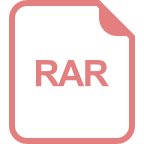
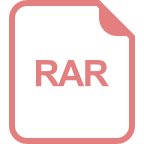
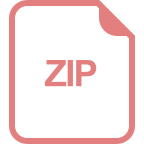
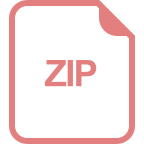
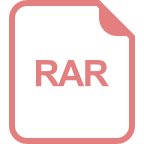
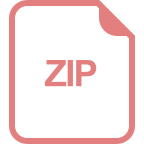
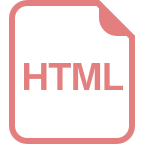
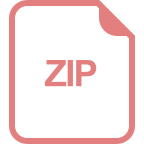
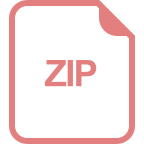
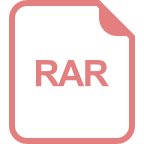
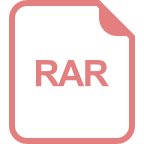
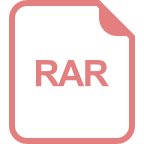