python爬虫,根据输入内容爬取相应图片,并显示相关进度,时间
时间: 2024-09-25 19:19:13 浏览: 78
Python爬虫结合了requests库用于发送HTTP请求获取网页内容,BeautifulSoup或 lxml 库解析HTML,以及PIL或io库处理下载图片。为了实现按输入内容抓取并显示进度和时间,你可以采用以下步骤:
1. **设置目标网站**:首先确定你要爬取的网站,了解其图片URL结构。
2. **用户输入处理**:创建一个函数接受用户输入的内容作为关键词,然后搜索该关键词在网页中的图片链接。
3. **爬虫逻辑**:
- 使用`requests.get()`获取网页源码。
- 使用BeautifulSoup解析HTML,找到包含图片的标签,如`<img>`元素,从中提取src属性作为链接。
- 对每个找到的链接,检查是否包含用户输入的关键字,如果匹配则保存到待下载列表中。
4. **下载图片并显示进度**:
- 可以利用`concurrent.futures`模块并发下载图片,提高效率。
- 创建一个计数器记录已下载的图片数,同时计算下载速度和总耗时。
- 每当下载完一张图片,更新进度信息,并显示当前时间和已用时间。
5. **进度展示**:
- 使用`time`模块来记录开始和结束时间,以及每次下载后的更新。
- 可以通过控制台输出或者GUI界面显示进度百分比、已经下载的图片数等信息。
6. **异常处理**:添加适当的错误处理,比如网络连接失败、下载中断等情况。
示例代码片段如下:
```python
import requests
from bs4 import BeautifulSoup
import time
from concurrent.futures import ThreadPoolExecutor
import os
def find_images(keyword):
url = 'http://example.com' # 替换为你想爬取的网站
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
images = soup.find_all('img', src=True) # 这里只是一个简化例子,实际需要根据网站结构调整
filtered_images = [img['src'] for img in images if keyword in img['src']]
return filtered_images
def download_image(image_url, output_dir):
try:
response = requests.get(image_url, stream=True)
filename = os.path.join(output_dir, os.path.basename(image_url))
with open(filename, 'wb') as f:
for chunk in response.iter_content(1024):
f.write(chunk)
except Exception as e:
print(f"Download failed: {e}")
def main(keyword, num_threads=5):
start_time = time.time()
images = find_images(keyword)
total_images = len(images)
output_dir = 'downloaded_images'
os.makedirs(output_dir, exist_ok=True)
with ThreadPoolExecutor(max_workers=num_threads) as executor:
future_to_url = {executor.submit(download_image, image, output_dir): image for image in images}
for future in concurrent.futures.as_completed(future_to_url):
try:
img_url = future_to_url[future]
percentage = (future.done() * 100) / total_images
print(f"Downloaded {percentage:.2f}% | Image URL: {img_url} | Elapsed Time: {time.strftime('%H:%M:%S', time.gmtime(time.time() - start_time))}")
except Exception as exc:
print(f"Error occurred: {exc}")
if __name__ == "__main__":
input_keyword = input("Enter the keyword to search for images: ")
main(input_keyword)
```
阅读全文
相关推荐
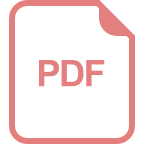
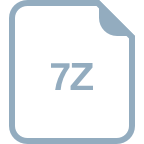
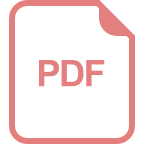
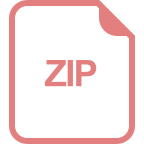
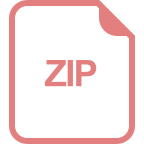
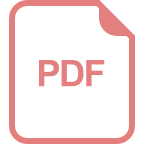
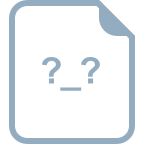
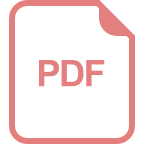
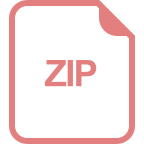
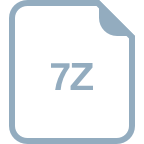
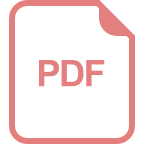
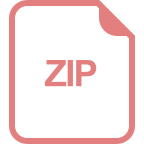
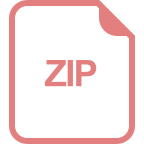
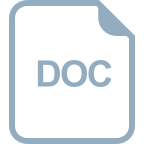
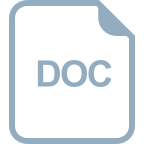
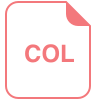
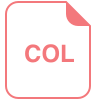
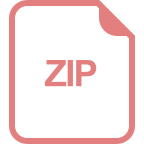